Describes a two dimensional icon located at the position of the containing
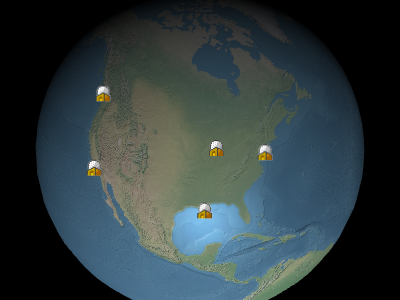
Example billboards
Entity
.
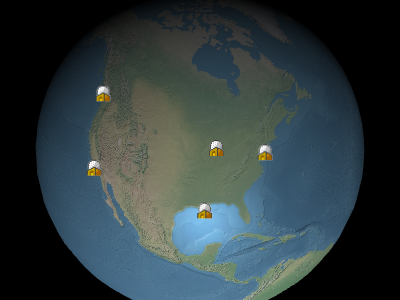
Example billboards
Name | Type | Description |
---|---|---|
options |
BillboardGraphics.ConstructorOptions | optional Object describing initialization options |
Members
alignedAxis : Property|undefined
Gets or sets the
Cartesian3
Property specifying the unit vector axis of rotation
in the fixed frame. When set to Cartesian3.ZERO the rotation is from the top of the screen.
-
Default Value:
Cartesian3.ZERO
color : Property|undefined
Gets or sets the Property specifying the
Color
that is multiplied with the image
.
This has two common use cases. First, the same white texture may be used by many different billboards,
each with a different color, to create colored billboards. Second, the color's alpha component can be
used to make the billboard translucent as shown below. An alpha of 0.0
makes the billboard
transparent, and 1.0
makes the billboard opaque.
default ![]() |
alpha : 0.5 ![]() |
-
Default Value:
Color.WHITE
readonly definitionChanged : Event
Gets the event that is raised whenever a property or sub-property is changed or modified.
disableDepthTestDistance : Property|undefined
Gets or sets the distance from the camera at which to disable the depth test to, for example, prevent clipping against terrain.
When set to zero, the depth test is always applied. When set to Number.POSITIVE_INFINITY, the depth test is never applied.
distanceDisplayCondition : Property|undefined
Gets or sets the
DistanceDisplayCondition
Property specifying at what distance from the camera that this billboard will be displayed.
eyeOffset : Property|undefined
Gets or sets the
Cartesian3
Property specifying the billboard's offset in eye coordinates.
Eye coordinates is a left-handed coordinate system, where x
points towards the viewer's
right, y
points up, and z
points into the screen.
An eye offset is commonly used to arrange multiple billboards or objects at the same position, e.g., to arrange a billboard above its corresponding 3D model.
Below, the billboard is positioned at the center of the Earth but an eye offset makes it always appear on top of the Earth regardless of the viewer's or Earth's orientation.
![]() |
![]() |
b.eyeOffset = new Cartesian3(0.0, 8000000.0, 0.0);
-
Default Value:
Cartesian3.ZERO
height : Property|undefined
Gets or sets the numeric Property specifying the height of the billboard in pixels.
When undefined, the native height is used.
heightReference : Property|undefined
Gets or sets the Property specifying the
HeightReference
.
-
Default Value:
HeightReference.NONE
horizontalOrigin : Property|undefined
Gets or sets the Property specifying the
HorizontalOrigin
.
-
Default Value:
HorizontalOrigin.CENTER
image : Property|undefined
Gets or sets the Property specifying the Image, URI, or Canvas to use for the billboard.
imageSubRegion : Property|undefined
Gets or sets the Property specifying a
BoundingRectangle
that defines a
sub-region of the image
to use for the billboard, rather than the entire image,
measured in pixels from the bottom-left.
pixelOffset : Property|undefined
Gets or sets the
The billboard's origin is indicated by the yellow point.
Cartesian2
Property specifying the billboard's pixel offset in screen space
from the origin of this billboard. This is commonly used to align multiple billboards and labels at
the same position, e.g., an image and text. The screen space origin is the top, left corner of the
canvas; x
increases from left to right, and y
increases from top to bottom.
default ![]() |
b.pixeloffset = new Cartesian2(50, 25); ![]() |
-
Default Value:
Cartesian2.ZERO
pixelOffsetScaleByDistance : Property|undefined
Gets or sets
NearFarScalar
Property specifying the pixel offset of the billboard based on the distance from the camera.
A billboard's pixel offset will interpolate between the NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's pixel offset remains clamped to the nearest bound.
rotation : Property|undefined
Gets or sets the numeric Property specifying the rotation of the image
counter clockwise from the
alignedAxis
.
-
Default Value:
0
scale : Property|undefined
Gets or sets the numeric Property specifying the uniform scale to apply to the image.
A scale greater than
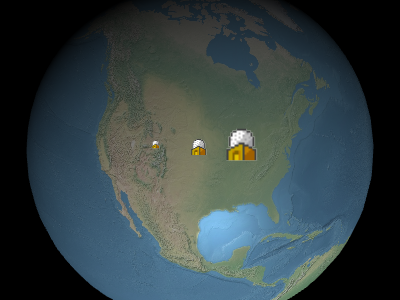
From left to right in the above image, the scales are
1.0
enlarges the billboard while a scale less than 1.0
shrinks it.
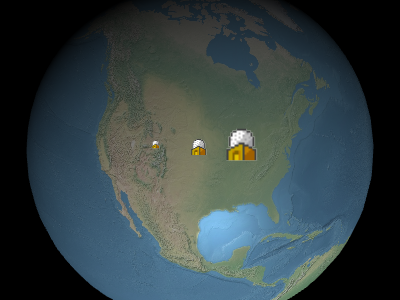
From left to right in the above image, the scales are
0.5
, 1.0
, and 2.0
.
-
Default Value:
1.0
scaleByDistance : Property|undefined
Gets or sets
NearFarScalar
Property specifying the scale of the billboard based on the distance from the camera.
A billboard's scale will interpolate between the NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's scale remains clamped to the nearest bound.
show : Property|undefined
Gets or sets the boolean Property specifying the visibility of the billboard.
-
Default Value:
true
sizeInMeters : Property|undefined
Gets or sets the boolean Property specifying if this billboard's size will be measured in meters.
-
Default Value:
false
splitDirection : Property|undefined
Gets or sets the Property specifying the
SplitDirection
of this billboard.
-
Default Value:
SplitDirection.NONE
translucencyByDistance : Property|undefined
Gets or sets
NearFarScalar
Property specifying the translucency of the billboard based on the distance from the camera.
A billboard's translucency will interpolate between the NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's translucency remains clamped to the nearest bound.
verticalOrigin : Property|undefined
Gets or sets the Property specifying the
VerticalOrigin
.
-
Default Value:
VerticalOrigin.CENTER
width : Property|undefined
Gets or sets the numeric Property specifying the width of the billboard in pixels.
When undefined, the native width is used.
Methods
clone(result) → BillboardGraphics
Duplicates this instance.
Name | Type | Description |
---|---|---|
result |
BillboardGraphics | optional The object onto which to store the result. |
Returns:
The modified result parameter or a new instance if one was not provided.
Assigns each unassigned property on this object to the value
of the same property on the provided source object.
Name | Type | Description |
---|---|---|
source |
BillboardGraphics | The object to be merged into this object. |
Type Definitions
Initialization options for the BillboardGraphics constructor
Properties:
Name | Type | Attributes | Default | Description |
---|---|---|---|---|
show |
Property | boolean |
<optional> |
true | A boolean Property specifying the visibility of the billboard. |
image |
Property | string | HTMLCanvasElement |
<optional> |
A Property specifying the Image, URI, or Canvas to use for the billboard. | |
scale |
Property | number |
<optional> |
1.0 | A numeric Property specifying the scale to apply to the image size. |
pixelOffset |
Property | Cartesian2 |
<optional> |
Cartesian2.ZERO | A Cartesian2 Property specifying the pixel offset. |
eyeOffset |
Property | Cartesian3 |
<optional> |
Cartesian3.ZERO | A Cartesian3 Property specifying the eye offset. |
horizontalOrigin |
Property | HorizontalOrigin |
<optional> |
HorizontalOrigin.CENTER | A Property specifying the HorizontalOrigin . |
verticalOrigin |
Property | VerticalOrigin |
<optional> |
VerticalOrigin.CENTER | A Property specifying the VerticalOrigin . |
heightReference |
Property | HeightReference |
<optional> |
HeightReference.NONE | A Property specifying what the height is relative to. |
color |
Property | Color |
<optional> |
Color.WHITE | A Property specifying the tint Color of the image. |
rotation |
Property | number |
<optional> |
0 | A numeric Property specifying the rotation about the alignedAxis. |
alignedAxis |
Property | Cartesian3 |
<optional> |
Cartesian3.ZERO | A Cartesian3 Property specifying the unit vector axis of rotation. |
sizeInMeters |
Property | boolean |
<optional> |
A boolean Property specifying whether this billboard's size should be measured in meters. | |
width |
Property | number |
<optional> |
A numeric Property specifying the width of the billboard in pixels, overriding the native size. | |
height |
Property | number |
<optional> |
A numeric Property specifying the height of the billboard in pixels, overriding the native size. | |
scaleByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to scale the point based on distance from the camera. |
|
translucencyByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to set translucency based on distance from the camera. |
|
pixelOffsetScaleByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to set pixelOffset based on distance from the camera. |
|
imageSubRegion |
Property | BoundingRectangle |
<optional> |
A Property specifying a BoundingRectangle that defines a sub-region of the image to use for the billboard, rather than the entire image, measured in pixels from the bottom-left. |
|
distanceDisplayCondition |
Property | DistanceDisplayCondition |
<optional> |
A Property specifying at what distance from the camera that this billboard will be displayed. | |
disableDepthTestDistance |
Property | number |
<optional> |
A Property specifying the distance from the camera at which to disable the depth test to. | |
splitDirection |
Property | SplitDirection |
<optional> |
A Property specifying the SplitDirection of the billboard. |