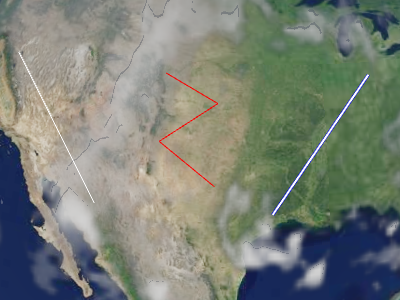
Example polylines
Polylines are added and removed from the collection using
PolylineCollection#add
and PolylineCollection#remove
.
Performance:
For best performance, prefer a few collections, each with many polylines, to many collections with only a few polylines each. Organize collections so that polylines with the same update frequency are in the same collection, i.e., polylines that do not change should be in one collection; polylines that change every frame should be in another collection; and so on.
Name | Type | Description | ||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
object |
optional
Object with the following properties:
|
Example:
// Create a polyline collection with two polylines
const polylines = new Cesium.PolylineCollection();
polylines.add({
positions : Cesium.Cartesian3.fromDegreesArray([
-75.10, 39.57,
-77.02, 38.53,
-80.50, 35.14,
-80.12, 25.46]),
width : 2
});
polylines.add({
positions : Cesium.Cartesian3.fromDegreesArray([
-73.10, 37.57,
-75.02, 36.53,
-78.50, 33.14,
-78.12, 23.46]),
width : 4
});
See:
Members
Draws the bounding sphere for each draw command in the primitive.
-
Default Value:
false
PolylineCollection#get
to iterate over all the polylines
in the collection.
modelMatrix : Matrix4
Transforms.eastNorthUpToFixedFrame
.
-
Default Value:
Matrix4.IDENTITY
-
Default Value:
true
Methods
add(options) → Polyline
Performance:
After calling add
, PolylineCollection#update
is called and
the collection's vertex buffer is rewritten - an O(n)
operation that also incurs CPU to GPU overhead.
For best performance, add as many polylines as possible before calling update
.
Name | Type | Description |
---|---|---|
options |
object | optional A template describing the polyline's properties as shown in Example 1. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Example 1: Add a polyline, specifying all the default values.
const p = polylines.add({
show : true,
positions : ellipsoid.cartographicArrayToCartesianArray([
Cesium.Cartographic.fromDegrees(-75.10, 39.57),
Cesium.Cartographic.fromDegrees(-77.02, 38.53)]),
width : 1
});
See:
Name | Type | Description |
---|---|---|
polyline |
Polyline | The polyline to check for. |
Returns:
Once an object is destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception. Therefore,
assign the return value (undefined
) to the object as done in the example.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
polylines = polylines && polylines.destroy();
See:
get(index) → Polyline
PolylineCollection#length
to iterate over all the polylines
in the collection.
Performance:
If polylines were removed from the collection and
PolylineCollection#update
was not called, an implicit O(n)
operation is performed.
Name | Type | Description |
---|---|---|
index |
number | The zero-based index of the polyline. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Toggle the show property of every polyline in the collection
const len = polylines.length;
for (let i = 0; i < len; ++i) {
const p = polylines.get(i);
p.show = !p.show;
}
See:
If this object was destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception.
Returns:
true
if this object was destroyed; otherwise, false
.
Performance:
After calling remove
, PolylineCollection#update
is called and
the collection's vertex buffer is rewritten - an O(n)
operation that also incurs CPU to GPU overhead.
For best performance, remove as many polylines as possible before calling update
.
If you intend to temporarily hide a polyline, it is usually more efficient to call
Polyline#show
instead of removing and re-adding the polyline.
Name | Type | Description |
---|---|---|
polyline |
Polyline | The polyline to remove. |
Returns:
true
if the polyline was removed; false
if the polyline was not found in the collection.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
const p = polylines.add(...);
polylines.remove(p); // Returns true
See:
Performance:
O(n)
. It is more efficient to remove all the polylines
from a collection and then add new ones than to create a new collection entirely.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
polylines.add(...);
polylines.add(...);
polylines.removeAll();
See:
Viewer
or CesiumWidget
render the scene to
get the draw commands needed to render this primitive.
Do not call this function directly. This is documented just to list the exceptions that may be propagated when the scene is rendered:
Throws:
-
RuntimeError : Vertex texture fetch support is required to render primitives with per-instance attributes. The maximum number of vertex texture image units must be greater than zero.