CesiumJS Quickstart
This is a quickstart to building 3D applications with Cesium using real-world data. You’ll learn to set up a Cesium app on a web page like this:
A basic Cesium app loading global 3D terrain and buildings in San Francisco. Click to interact, or type an address in the search box.
Cesium ion is an open platform for streaming and hosting 3D content.
Sign up for a free Cesium ion account to get global satellite imagery and real-world 3D content for your app.
Once you’re logged in:
- Go to your Access Tokens tab.
- Note the copy button next to the default token. We’ll use this token in the next step.
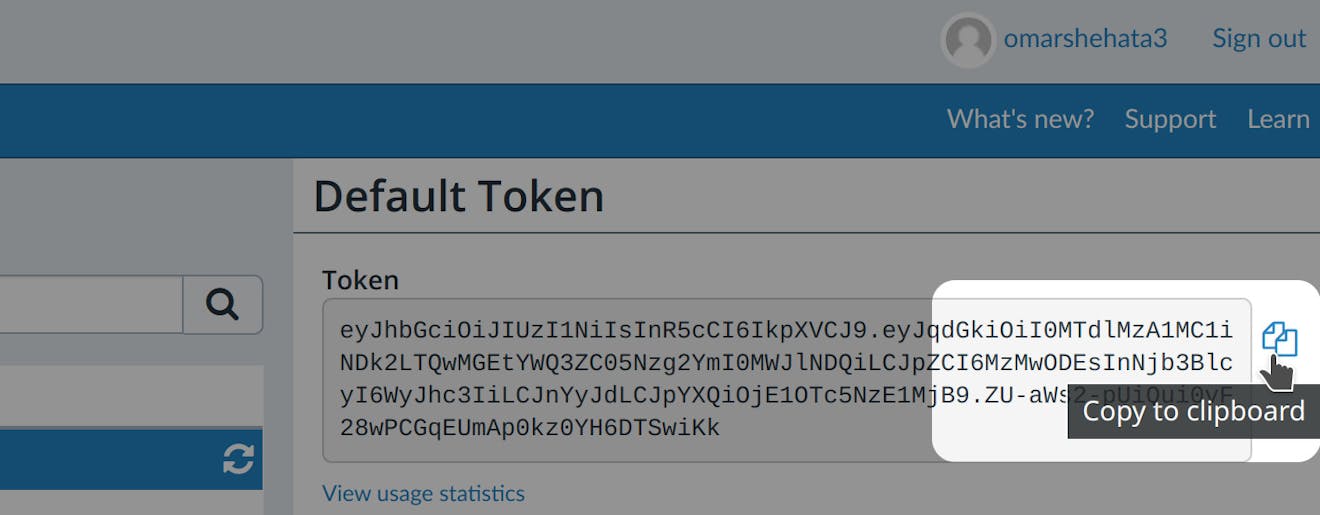
CesiumJS is an open source JavaScript engine. We’ll use it to visualize the content we load from Cesium ion.
This guide covers two ways to set up CesiumJS:
- Import from CDN
- Install with NPM
Import from CDN
Below is a complete HTML page that will load the required JavaScript and CSS files and initialize the scene at San Francisco. If you don't have a development environment, you can create a file containing this HTML and view it in a browser.
Just replace your_access_token
with your Cesium ion access token.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<!-- Include the CesiumJS JavaScript and CSS files -->
<script src="https://cesium.com/downloads/cesiumjs/releases/1.116/Build/Cesium/Cesium.js"></script>
<link href="https://cesium.com/downloads/cesiumjs/releases/1.116/Build/Cesium/Widgets/widgets.css" rel="stylesheet">
</head>
<body>
<div id="cesiumContainer"></div>
<script type="module">
// Your access token can be found at: https://ion.cesium.com/tokens.
// Replace `your_access_token` with your Cesium ion access token.
Cesium.Ion.defaultAccessToken = 'your_access_token';
// Initialize the Cesium Viewer in the HTML element with the `cesiumContainer` ID.
const viewer = new Cesium.Viewer('cesiumContainer', {
terrain: Cesium.Terrain.fromWorldTerrain(),
});
// Fly the camera to San Francisco at the given longitude, latitude, and height.
viewer.camera.flyTo({
destination: Cesium.Cartesian3.fromDegrees(-122.4175, 37.655, 400),
orientation: {
heading: Cesium.Math.toRadians(0.0),
pitch: Cesium.Math.toRadians(-15.0),
}
});
// Add Cesium OSM Buildings, a global 3D buildings layer.
const buildingTileset = await Cesium.createOsmBuildingsAsync();
viewer.scene.primitives.add(buildingTileset);
</script>
</div>
</body>
</html>
The code in the Sandcastle is slightly different because Sandcastle doesn't require your token to authenticate and because it automatically creates the high-level HTML tags.
npm install cesium
The code below loads the required JavaScript and CSS files.
Just replace your_access_token
with your Cesium ion access token.
// The URL on your server where CesiumJS's static files are hosted.
window.CESIUM_BASE_URL = '/';
import { Cartesian3, createOsmBuildingsAsync, Ion, Math as CesiumMath, Terrain, Viewer } from 'cesium';
import "cesium/Build/Cesium/Widgets/widgets.css";
// Your access token can be found at: https://ion.cesium.com/tokens.
// Replace `your_access_token` with your Cesium ion access token.
Ion.defaultAccessToken = 'your_access_token';
// Initialize the Cesium Viewer in the HTML element with the `cesiumContainer` ID.
const viewer = new Viewer('cesiumContainer', {
terrain: Terrain.fromWorldTerrain(),
});
// Fly the camera to San Francisco at the given longitude, latitude, and height.
viewer.camera.flyTo({
destination: Cartesian3.fromDegrees(-122.4175, 37.655, 400),
orientation: {
heading: CesiumMath.toRadians(0.0),
pitch: CesiumMath.toRadians(-15.0),
}
});
// Add Cesium OSM Buildings, a global 3D buildings layer.
const buildingTileset = await createOsmBuildingsAsync();
viewer.scene.primitives.add(buildingTileset);
Configuring CESIUM_BASE_URL
CesiumJS requires a few static files to be hosted on your server, like web workers and SVG icons. Configure your module bundler to copy the following four directories and serve them as static files:
node_modules/cesium/Build/Cesium/Workers
node_modules/cesium/Build/Cesium/ThirdParty
node_modules/cesium/Build/Cesium/Assets
node_modules/cesium/Build/Cesium/Widgets
The window.CESIUM_BASE_URL
global variable must be set before CesiumJS is imported. It must point to the URL where those four directories are served.
For example, if the image at Assets/Images/cesium_credit.png
is served with a static/Cesium/
prefix under http://localhost:8080/static/Cesium/Assets/Images/cesium_credit.png
, then you would set the base URL as follows:
window.CESIUM_BASE_URL = '/static/Cesium/';
See the Cesium Webpack Example for a complete Webpack config.
Now that you’ve set up your Cesium application, there’s a whole world to explore! See what you can build with these tutorials:
Build a Flight Tracker: visualize a real flight from San Francisco to Copenhagen with radar data collected by FlightRadar24.
Visualize a Proposed Building: replace a building in a real city with your own 3D model and see how it changes the view.