Control the camera
This guide will show you how to work with the camera API in CesiumJS and implement functions like fly to a point on earth, zoom to a data source in the scene, or lock the camera to a specific model.
- Know how to set up a basic CesiumJS application.
New to Cesium? For help setting up an app for the first time, start with the Quickstart tutorial.
If you know the latitude, longitude, and height of the location, you can use the camera’s flyTo
function to fly directly to that location in CesiumJS. For instance, to fly to San Diego, we pass in -117.16, 32.71, and 15000.0 for longitude, latitude, and height respectively:
viewer.camera.flyTo({
destination: Cesium.Cartesian3.fromDegrees(-117.16, 32.71, 15000.0),
});
To change the orientation of the camera once flyTo
has completed, add an orientation
option:
viewer.camera.flyTo({
destination: Cesium.Cartesian3.fromDegrees(-117.16, 32.71, 15000.0),
orientation: {
heading: Cesium.Math.toRadians(20.0),
pitch: Cesium.Math.toRadians(-35.0),
roll: 0.0,
},
});
The heading, pitch, and roll here are relative to East, North, and Up respectively.
Get location of a point
If you know the name of the location you want to fly to but are not sure what the coordinates of that location is, you can use pickPosition
to find out. The following code snippet will print out the location of a point in Cartesian3
on click:
var handler = new Cesium.ScreenSpaceEventHandler(viewer.canvas);
handler.setInputAction(function (event) {
var pickedPosition = viewer.scene.pickPosition(event.position);
if (Cesium.defined(pickedPosition)) {
console.log(pickedPosition);
}
}, Cesium.ScreenSpaceEventType.LEFT_CLICK);
If you have added an asset to your scene and want to focus the camera on it, you can use viewer’s flyTo
. The viewer.flyTo
function takes in an Entity
, EntityCollection
, DataSource
, Cesium3DTilset
, and much more.
For example, focus on a rectangle Entity
that has been added to the scene with this code snippet:
var west = -90.0;
var south = 38.0;
var east = -87.0;
var north = 40.0;
var rectangle = viewer.entities.add({
rectangle: {
coordinates: Cesium.Rectangle.fromDegrees(west, south, east, north),
},
});
viewer.flyTo(rectangle);
An option to the camera.flyTo
function is easingFunction
. An easing function controls how the time is interpolated during a camera flight duration. The following code example sets the easing function to QUADRATIC_IN_OUT
to fly from the Tokyo Skytree to the Seattle Space Needle:
viewer.camera.flyTo({
destination: new Cesium.Cartesian3(
-3961951.575572026,
3346492.0945766014,
3702340.5336036095
),
orientation: {
direction: new Cesium.Cartesian3(
0.8982074415844437,
-0.4393530288745287,
0.013867512433959908
),
up: new Cesium.Cartesian3(
0.12793638617798253,
0.29147314437764565,
0.9479850669701113
),
},
complete: function () {
setTimeout(function () {
viewer.camera.flyTo({
destination: new Cesium.Cartesian3(
-2304817.2435183465,
-3639113.128132953,
4688495.013644141
),
orientation: {
direction: new Cesium.Cartesian3(
0.3760550186878076,
0.9007147395506565,
0.21747547189489164
),
up: new Cesium.Cartesian3(
-0.20364591529594356,
-0.14862471084230877,
0.9676978022659334
),
},
easingFunction: Cesium.EasingFunction.QUADRATIC_IN_OUT,
duration: 5,
});
}, 1000);
},
});
Beside flying to a point on the globe, you can also lock the camera to a point. This comes in handy when you want to restrict the camera’s movements to a region or asset.
To lock the camera to a point, use the camera’s lookAtTransform
function. The following code snippet locks the camera to Mount Fuji, Japan:
const center = Cesium.Cartesian3.fromRadians(
2.4213211833389243,
0.6171926869414084,
3626.0426275055174
);
const transform = Cesium.Transforms.eastNorthUpToFixedFrame(center);
viewer.scene.camera.lookAtTransform(
transform,
new Cesium.HeadingPitchRange(0, -Math.PI / 4, 2900)
);
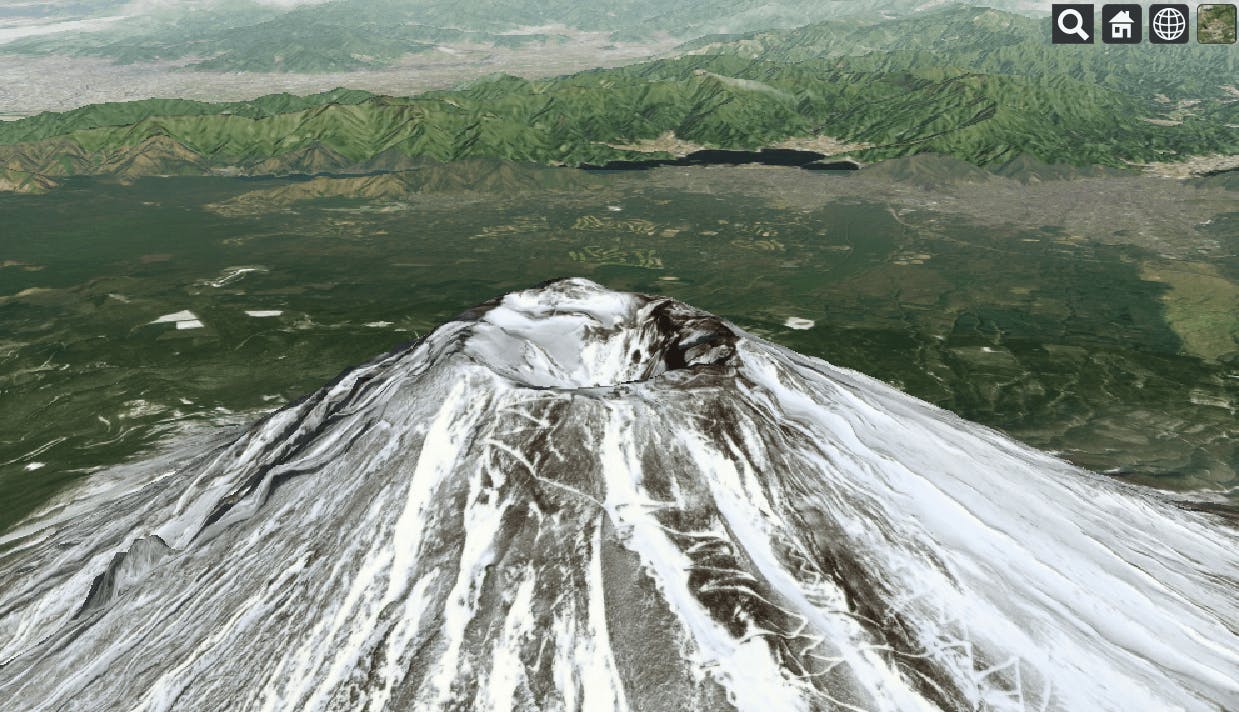
Once you have the camera locked to a point, you can create a camera orbit to showcase your asset. This can be achieved by using the camera’s lookAtTransform
function with an event listener:
// Lock camera to a point
const center = Cesium.Cartesian3.fromRadians(
2.4213211833389243,
0.6171926869414084,
3626.0426275055174
);
const transform = Cesium.Transforms.eastNorthUpToFixedFrame(center);
viewer.scene.camera.lookAtTransform(
transform,
new Cesium.HeadingPitchRange(0, -Math.PI / 8, 2900)
);
// Orbit this point
viewer.clock.onTick.addEventListener(function (clock) {
viewer.scene.camera.rotateRight(0.005);
});
The ScreenSpaceCameraController
converts user input, such as mouse and touch, from window coordinates to camera motion. It contains properties for enabling and disabling different types of input, modifying the amount of inertia and the minimum and maximum zoom distances.
For instance, you can use the ScreenSpaceCameraController
to control whether the camera is allowed to go underground:
// Disable camera collision to allow it to go underground
viewer.scene.screenSpaceCameraController.enableCollisionDetection = false;
Check out the camera examples in Sandcastle:
- Camera Tutorial—code example of camera movements.
- Camera—code examples to fly to locations, view extents, and set the camera’s reference frame.
Also, check out the reference documentation: