Styling and Filtering 3D Tiles
This guide explains how to use CesiumJS to style and filter 3D Tiles to highlight important features of your dataset.
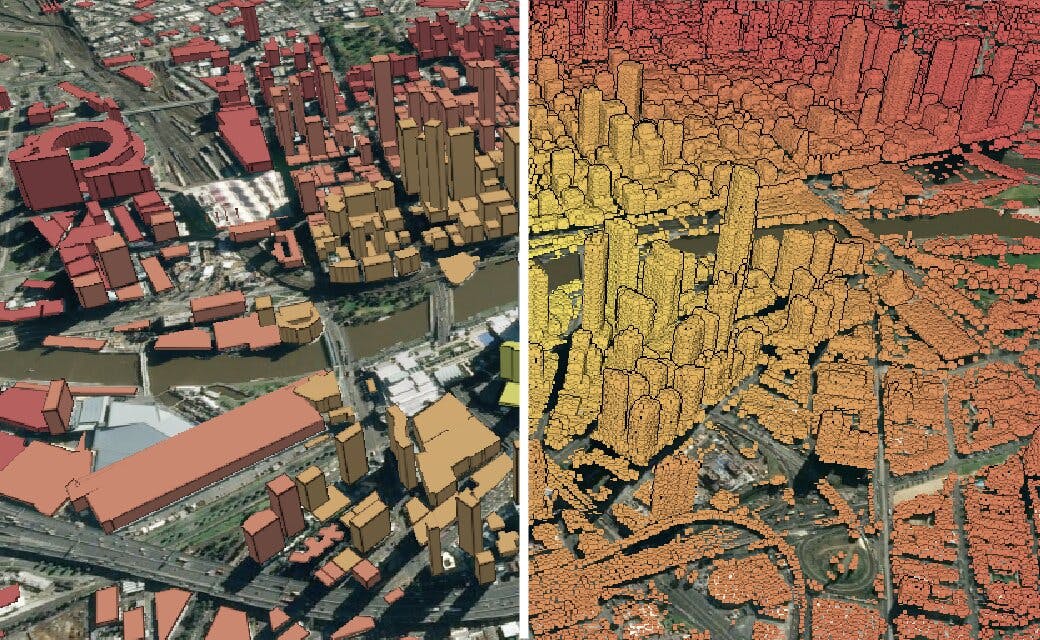
Heatmap of Melbourne created with 3D Tiles Styling. Left: OSM Buildings 3D Tiles. Right: Point cloud 3D Tiles.
- A 3D Tileset with properties or classification. You can upload your own data or use one of Cesium’s curated 3D Tilesets such as OSM Buildings or Montreal Point Cloud, which can be found in the Cesium ion Asset Depot. To turn your dataset into 3D Tiles, check out our quick guides for importing data.
Cesium ion’s tiling pipeline currently supports per-feature data for 3D buildings and point clouds, and we’re working on adding more.
- Basic knowledge about CesiumJS. For beginners, visit the Quickstart tutorial.
- A Cesium ion account to generate an access token for JSFiddle code examples (Step 1 of the Quickstart).
The 3D Tiles styling language lets you define rules for how features in your dataset should appear, for instance the color of buildings or the types of building that are shown. This is helpful for identifying patterns in a city or to create a more informative visualization.
Styles are defined with JSON and JavaScript expressions. Additionally, the styling language provides a set of built-in functions
to support common math operations, such as min
, distance
, and log
.
This guide includes examples of these commonly used parts of the 3D Tiles styling language:
- Styling options
show
andcolor
- Tests for
conditions
, including feature property tests, null check, andClassification
checks for points in point clouds - Creating new variables with
defines
- The
distance
function - Creating new color definitions
For in-depth documentation for styling and filtering 3D Tiles, see the 3D Tiles Styling Specification. More examples are also available in Sandcastle (search for “styling”).
What is a 3D Tiles feature?
Features can be things like individual buildings, doors, windows, valves, or points in a point cloud.
There are two common ways we can apply styles to a 3D tileset: via the show
and color visual properties. Once we have determined what the styling rule is, we can apply it to our features by setting the style
member variable of the 3D tileset to a new Cesium3DTileStyle.
For the code snippet below, we use the Cesium OSM Buildings 3D Tiles and the Crown Entertainment Complex in Melbourne, Australia. The rule is to color the building named “Crown Entertainment Complex” red and all other buildings white. CesiumJS will efficiently apply this rule to every feature, replacing any existing styles on the feature. The feature property we chose is name
, which already exists in the dataset.
osmBuildingsTileset.style = new Cesium.Cesium3DTileStyle({
color: {
conditions: [
["${name} === 'Crown Entertainment Complex'", "color('red')"],
["true", "color('white')"],
],
},
});
We can see a list of feature properties by clicking on a feature in the viewer:
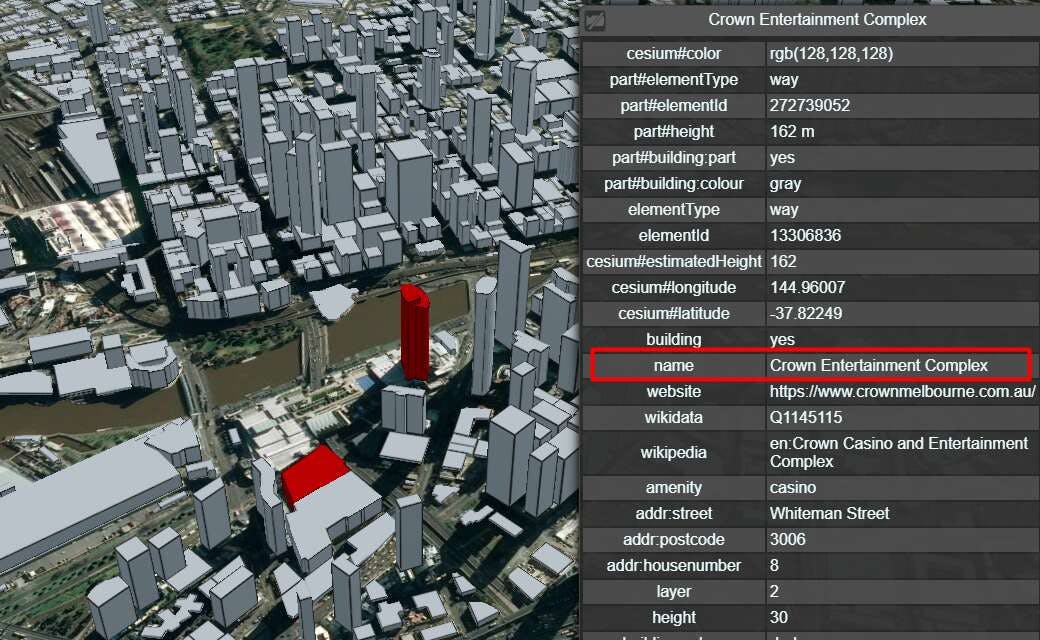
In addition to coloring, the 3D Tiles styling language allows you to show and hide features based on their metadata properties.
Here are all the buildings in Melbourne from the Cesium OSM Buildings tileset.
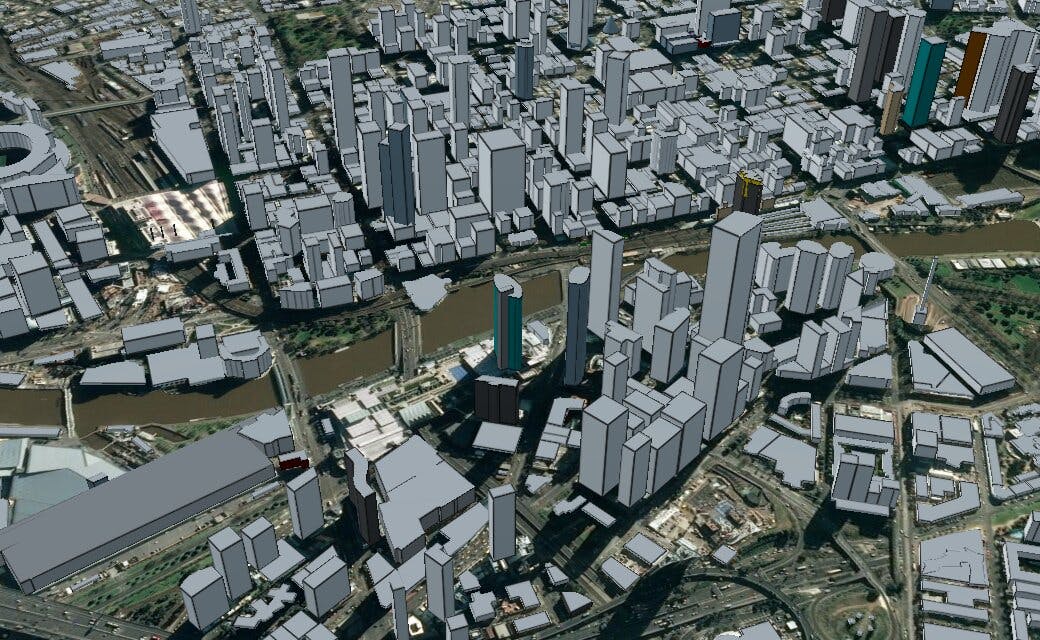
Melbourne prior to showing only residential buildings.
Let’s say we wanted to find all the residential buildings in Melbourne, which are classified using the building
property as “residential” or “apartment” in OSM Buildings.
osmBuildingsTileset.style = new Cesium.Cesium3DTileStyle({
show: "${feature['building']} === 'residential' || ${feature['building']} === 'apartments'",
});
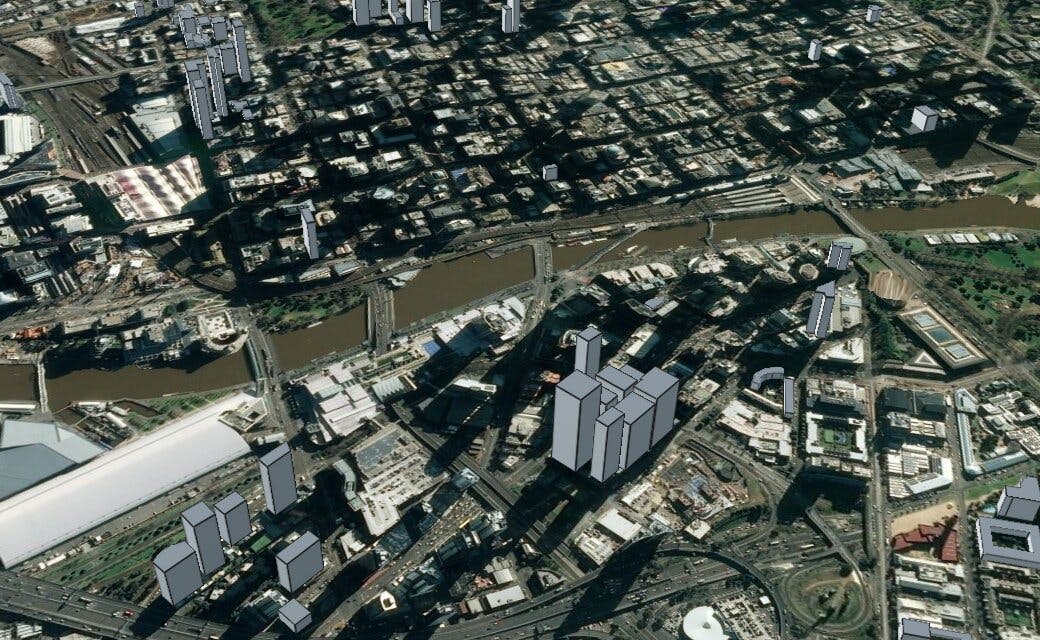
Melbourne showing only residential buildings.
You can also use conditions to determine what color to assign to features.
For example, you might want to design a bus route for tourists in a zone around Melbourne’s Crown Entertainment Complex. What buildings are within a given distance from the complex?
This code snippet assigns each building a color based on its distance from the Crown Entertainment Complex:
osmBuildingsTileset.style = new Cesium.Cesium3DTileStyle({
defines: {
distanceFromComplex:
"distance(vec2(${feature['cesium#longitude']}, ${feature['cesium#latitude']}), vec2(144.96007, -37.82249))",
},
color: {
conditions: [
["${distanceFromComplex} > 0.010", "color('#d65c5c')"],
["${distanceFromComplex} > 0.006", "color('#f58971')"],
["${distanceFromComplex} > 0.002", "color('#f5af71')"],
["${distanceFromComplex} > 0.0001", "color('#f5ec71')"],
["true", "color('#ffffff')"],
],
},
});
- 3D Tiles styling
conditions
lets you define features likecolor
in an array. - Conditions behave like if statements.
undefined - The
defines
expression creates new variables.
undefined distance
is abuilt-in function
of the 3D Tiles styling language.- CSS-style
color definitions
are supported.
undefined
The code snippet above produces the following styling result:
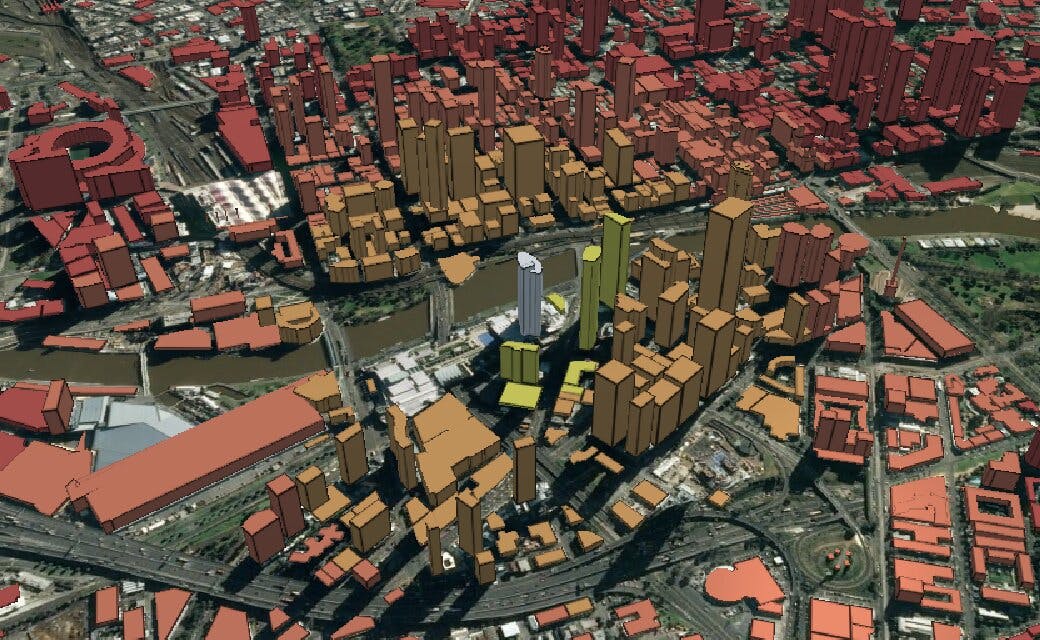
A point cloud is a collection of points that follow the same styling rules above, with some additional options.
Point cloud styling options and properties
In addition to color
and show
visual properties, point cloud Tiles also support pointSize
. The default pointSize
is 1.0.
Point clouds in LAS files tiled on Cesium ion include two per-point properties: Classification
and Intensity
.
Classification
is an integer mapping to the point’s data type, such as ground, low vegetation, or buildings.Intensity
is the reflectivity of the surface of your point, which is an integer between 0 and 255 inclusive.
Point cloud styling can also be applied to other point properties such as position, color, and normal. For instance, you can create a heatmap of point cloud tiles based on their distance from a central point using the POSITION_ABSOLUTE
metadata property, similar to how we colored OSM buildings based on their distance from the Crown Entertainment Complex (see the heatmap Sandcastle).
Point cloud styling example
Let’s say you want to visualize the environment surrounding the Biosphere Museum in Montreal, Canada. The styling rule is to color points green if their Classification
property is vegetation.
pointCloudTileset.style = new Cesium.Cesium3DTileStyle({
color: {
conditions: [
["${Classification} === 2", "color('brown')"], // ground
["${Classification} === 3", "color('greenyellow')"], // low vegetation
["${Classification} === 4", "color('green')"], // medium vegetation
["${Classification} === 5", "color('darkgreen')"], // high vegetation
["true", "color('white')"]
]
}
});
With the styling rule applied, we can visualize how much of the museum’s surroundings are vegetation:
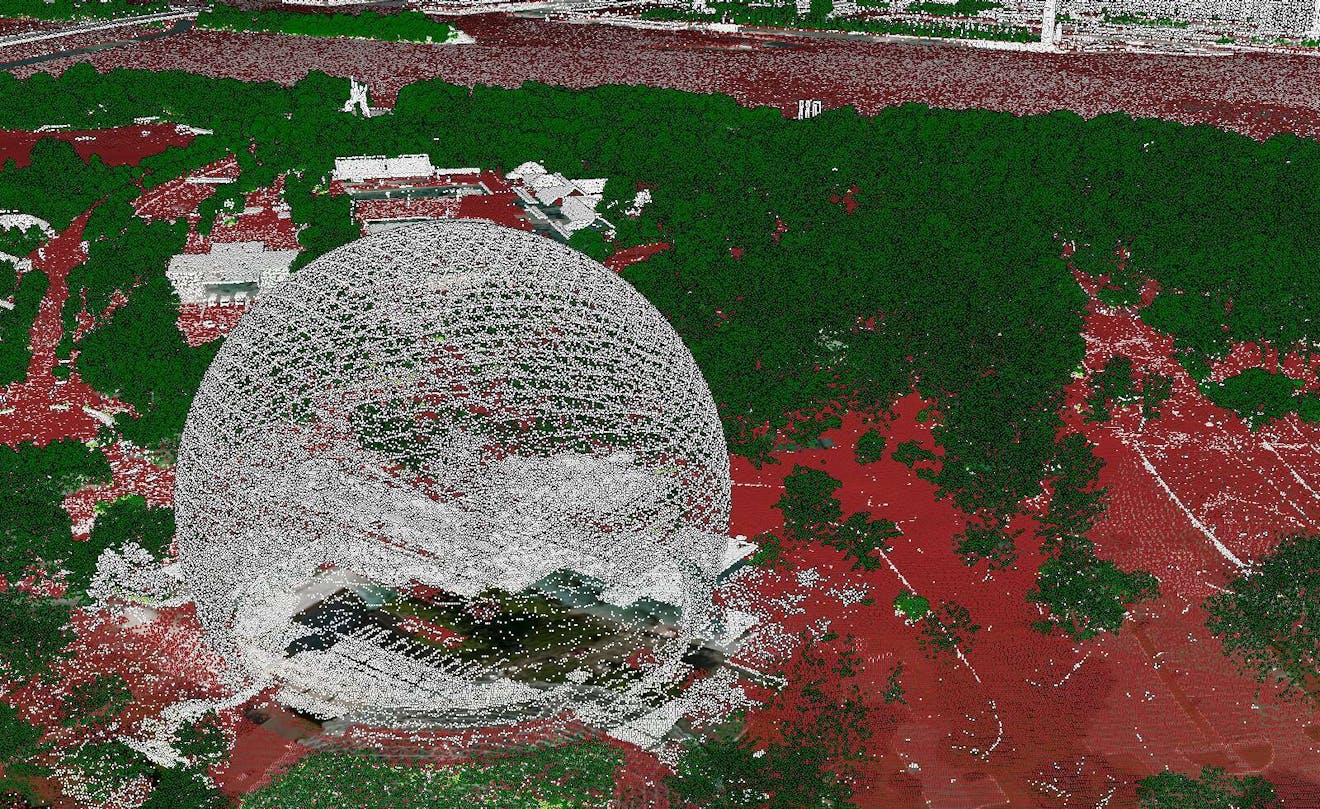
Sometimes buildings are missing properties. In such cases, we can add a null
checker condition:
osmBuildingsTileset.style = new Cesium.Cesium3DTileStyle({
color: {
conditions: [
["${material} === null", "color('white')"],
["${material} === 'glass'", "color('skyblue', 0.5)"],
["${material} === 'concrete'", "color('grey')"],
["${material} === 'brick'", "color('indianred')"],
["true", "color('white')"],
],
},
});
}
In this example, if any building doesn’t possess the material metadata property, its color is white.
You can also style 3D Tiles using the styling editor in Cesium Stories. The same styling methods we covered above can be applied in the editor without having to write code. See the Styling 3D Tiles in Cesium Stories tutorial.
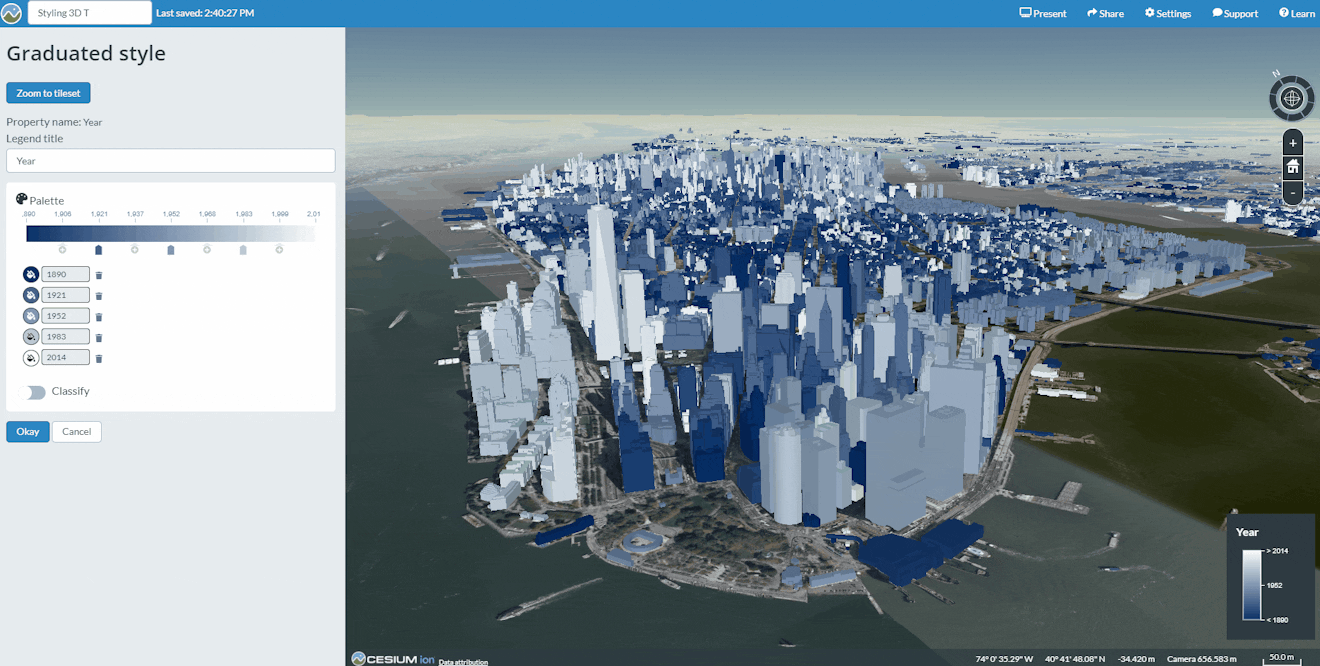
Check out the styling examples in Sandcastle, including:
- 3D Tiles Point Cloud Styling—code example for creating heatmap for point cloud 3D Tiles.
- Cesium OSM Buildings Styling—code example for styling OSM Buildings.
For in-depth documentation for styling and filtering 3D Tiles, see the 3D Tiles Styling Specification.