Configuring Vite or Webpack for CesiumJS
The CesiumJS library is compatible with all build systems and frameworks. However, given the nature of a rendering engine, there are a few extra steps you'll need to take to configure it for your application when using Vite or webpack.
We created a new example repository for using Cesium in Vite. We've recently seen a lot of questions about using Cesium in a Vite/Vue project, so we targeted Vite directly to cover the widest range of scenarios. We also updated our webpack example repository: it now contains examples for both webpack 4 and webpack 5.
Vite supports many different UI frameworks. We chose to target a Vite project with Vanilla JS, meaning no specific framework, as it's the lowest common denominator among all projects. The same configuration should apply to any Vite project.
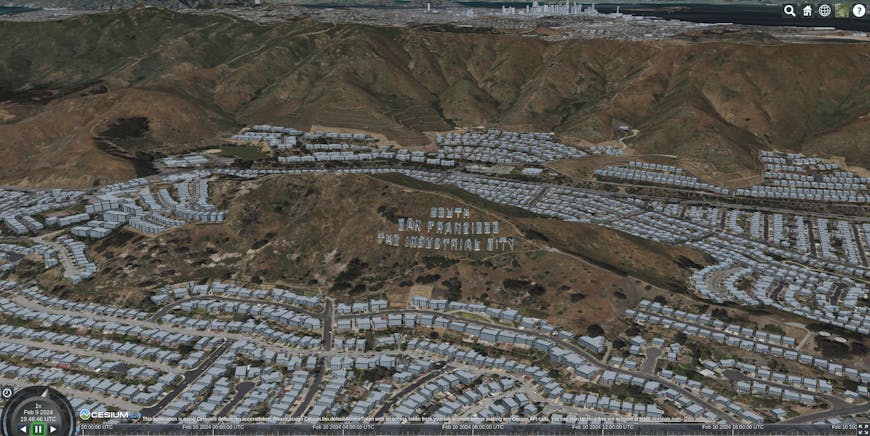
South San Francisco, California, USA, as shown when loading the sample Vite and webpack projects in CesiumJS.
Using Cesium in any build system
There are two things you need to do to incorporate Cesium into your project beyond including the npm package; this is covered in our quickstart guide.
- Include the Cesium Widgets CSS.
- Provide access to static files from the CesiumJS library (this includes the pre-built workers and other non-JS assets).
As of CesiumJS version 1.114, we’ve made improvements to the library so that it is no longer necessary to declare a few node packages as external. (See #11773.)
import "cesium/Build/Cesium/Widgets/widgets.css";
Vite can handle this by default, but webpack will need to define a loader for CSS. If you already have a webpack project, it is very likely this is already set up. If not, include the CSS loaders:
module: {
rules: [
// ...
{
test: /\.css/,
use: ["style-loader", "css-loader"],
},
// ...
]
}
Static files
There are 4 directories of static files that need to be included in your build:
node_modules/cesium/Build/Cesium/Workers
node_modules/cesium/Build/Cesium/ThirdParty
node_modules/cesium/Build/Cesium/Assets
node_modules/cesium/Build/Cesium/Widgets
In webpack (4 or 5), these can be included with the CopyWebpackPlugin
like this:
const cesiumSource = "node_modules/cesium/Build/Cesium";
const cesiumBaseUrl = "cesiumStatic";
// ...
new CopyWebpackPlugin({
patterns: [
{ from: path.join(cesiumSource, "Workers"), to: `${cesiumBaseUrl}/Workers`, },
{ from: path.join(cesiumSource, "ThirdParty"), to: `${cesiumBaseUrl}/ThirdParty`, },
{ from: path.join(cesiumSource, "Assets"), to: `${cesiumBaseUrl}/Assets`, },
{ from: path.join(cesiumSource, "Widgets"), to: `${cesiumBaseUrl}/Widgets`, },
],
}),
// ...
To achieve the same thing in Vite we use the viteStaticCopy
plugin:
const cesiumSource = "node_modules/cesium/Build/Cesium";
const cesiumBaseUrl = "cesiumStatic";
// ...
viteStaticCopy({
targets: [
{ src: `${cesiumSource}/ThirdParty`, dest: cesiumBaseUrl },
{ src: `${cesiumSource}/Workers`, dest: cesiumBaseUrl },
{ src: `${cesiumSource}/Assets`, dest: cesiumBaseUrl },
{ src: `${cesiumSource}/Widgets`, dest: cesiumBaseUrl },
],
}),
// ...
The cesiumBaseUrl
can be set to wherever you want CesiumJS asset files to be located in your build directory. However, you need to make sure to set the global window.CESIUM_BASE_URL
variable to that path so the CesiumJS code can access the files it needs.
In webpack we use the DefinePlugin
for this:
// ...
new webpack.DefinePlugin({
// Define relative base path in cesium for loading assets
CESIUM_BASE_URL: JSON.stringify(cesiumBaseUrl),
}),
// ...
In Vite we use the define
property of the defineConfig
options:
// ...
define: {
CESIUM_BASE_URL: JSON.stringify(cesiumBaseUrl),
},
// ...
We hope these updated examples make it easier for anyone to start using CesiumJS in their projects. Peruse the Vite and webpack repositories, and share your feedback on the community forum. We look forward to seeing what you build!