Migrating from Google Earth API Part II
This page is archived. Information on this page may be outdated. For the most recent tutorials and documentation, visit the CesiumJS Learning Center.
This tutorial looks at major concepts in CesiumJS from the perspective of a Google Earth plugin developer. This is Part II in a series of tutorials. If you haven’t already, check out Part I.
Positions and rectangles
When specifying positions, there are a few basic differences between the GE API and Cesium:
- GE uses latitude and longitude, in that order, and defines them in degrees. Natively, Cesium uses Cartesian positions (x, y, z) in WGS84 coordinates (and other reference frames like north-east-up).
- Cesium provides helper functions to construct a Cartesian position from longitude, latitude, and an optional height (in that order) like
Cartesian3.fromDegrees
andCartesian3.fromDegreesArray
. - With the exception of
fromDegrees
helpers, angles in Cesium are measured in radians, not degrees like GE. Like GE, distances are always in meters. - In GE, rectangle parameters are ordered north, south, east, west. In Cesium, they are west, south, east, north (think lower-left then upper-right).
Putting these concepts together, here’s an example of creating a ground overlay in Cesium:
var latitude = 40.0;
var longitude = -100.0;
var north = latitude + .35;
var south = latitude - .35;
var east = longitude + 1.55;
var west = longitude - 1.55;
var rectangle = Cesium.Rectangle.fromDegrees(west, south, east, north);
var entity = viewer.entities.add({
rectangle : {
coordinates : rectangle,
material : './static/Cesium_Logo_Color.jpg'
}
});
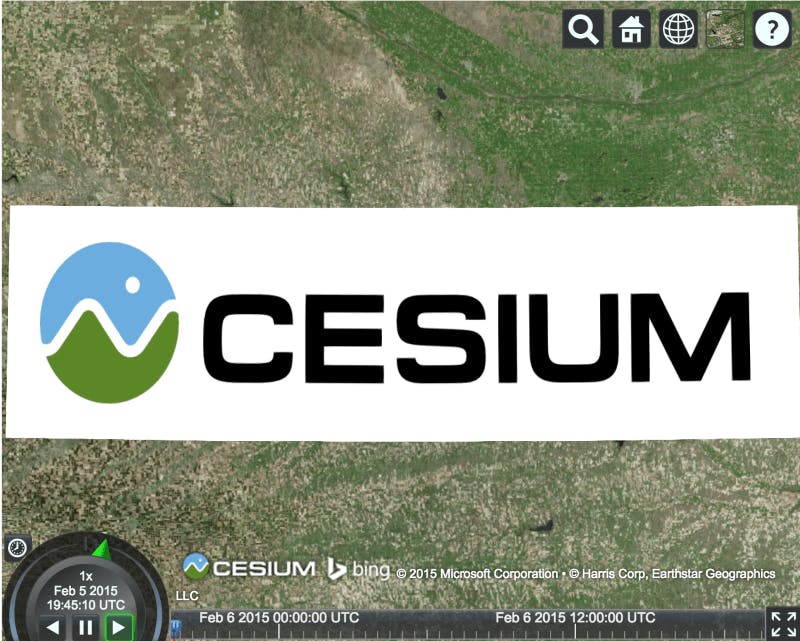
In general, the GE plugin API follows the layout of KML. The CesiumJS API is more general; it abstracts geospatial and graphics concepts, and provides more fine-grained control over the scene.
In the code above, we added an Entity which can contain different types of graphics…
Placemarks and beyond
In Cesium, an entity can represent a placemark by including a label
to represent the text and a billboard
to represent the icon. For example:
var pinBuilder = new Cesium.PinBuilder();
var entity = viewer.entities.add({
position : Cesium.Cartesian3.fromDegrees(-75.10, 39.57),
label : {
text : 'pin' + counter,
verticalOrigin : Cesium.VerticalOrigin.TOP
},
billboard : {
image : pinBuilder.fromColor(Cesium.Color.SALMON, 48).toDataURL(),
verticalOrigin : Cesium.VerticalOrigin.BOTTOM,
}
});
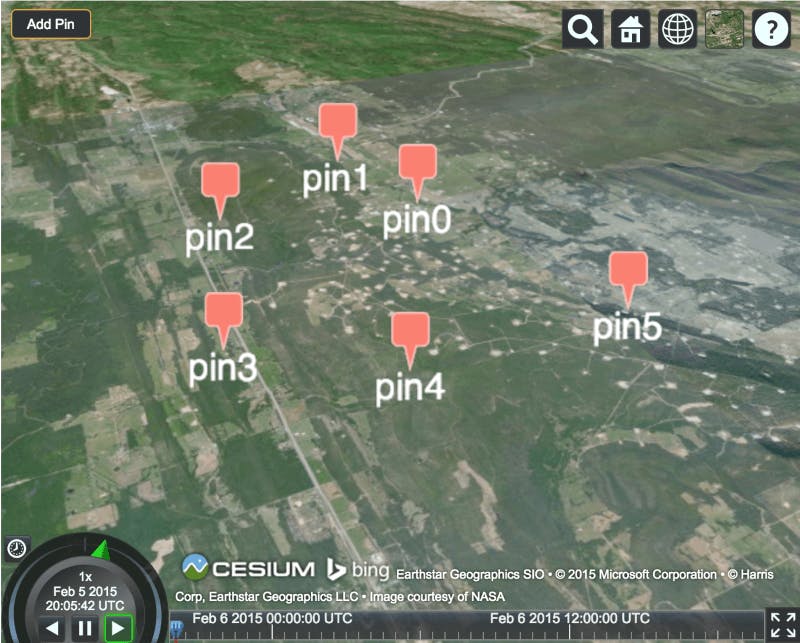
By default, the label
and billboard
are both centered at the entity’s position so the verticalOrigin
property is used to draw the label below the center point and the billboard above it.
In addition to assigning an image url to a billboard’s image, PinBuilder can be used to create custom pins.
Cesium offers many more shapes and volumes, such as cylinders, corridors, and ellipsoids. See the Creating Entties tutorial for a full list.
Camera control
Cesium has camera control similar to GE’s. Here’s a GE example:
var camera = ge.getView().copyAsCamera(ge.ALTITUDE_RELATIVE_TO_GROUND);
camera.set(latitude, longitude, altitude, ge.ALTITUDE_ABSOLUTE, heading, tilt, roll);
ge.getView().setAbstractView(camera);
In Cesium, this would be:
viewer.camera.setView({
destination : Cesium.Cartesian3.fromDegrees(longitude, latitude, altitude),
orientation: {
heading : Cesium.Math.toRadians(heading),
pitch : Cesium.Math.toRadians(tilt - 90.0),
roll : Cesium.Math.toRadians(roll)
}
});
The position, in degrees, is converted to Cartesian. The heading, pitch, and roll are converted from degrees to radians. In GE, tilt ranges from 0 (straight down) to 180 (straight up) compared to -90 (straight down) to 90 (straight up) so 90 degrees is subtracted.
viewer.camera.flyTo({
destination : Cesium.Cartesian3.fromDegrees(-75.1641667, 39.9522222, 6000.0),
orientation : {
heading : Cesium.Math.toRadians(35.0),
pitch : Cesium.Math.toRadians(-35.0),
roll : 0.0
},
duration : 4.0, // in seconds
complete : function() {
// called when the flight finishes
}
});
Since we often want to use the camera to view an entity, camera-entity integration allows us to easily zoom or fly to an entity or collection of entities:
var heading = Cesium.Math.toRadians(90);
var pitch = Cesium.Math.toRadians(-30);
viewer.zoomTo(entity, new Cesium.HeadingPitchRange(heading, pitch));
3D models
GE supports 3D models using COLLADA. Cesium supports 3D models, including key-frame animation, skinning, and individual node picking, using glTF, an emerging industry-standard format for 3D models on the web by the Khronos Group, the consortium behind WebGL and COLLADA.
glTF models have a .gltf
or .glb
extension and are loaded using the model
property of an entity.
var entity = viewer.entities.add({
position : Cesium.Cartesian3.fromDegrees(-100, 40),
model : {
uri : './static/Cesium_Man.gltf',
scale : 10
}
});
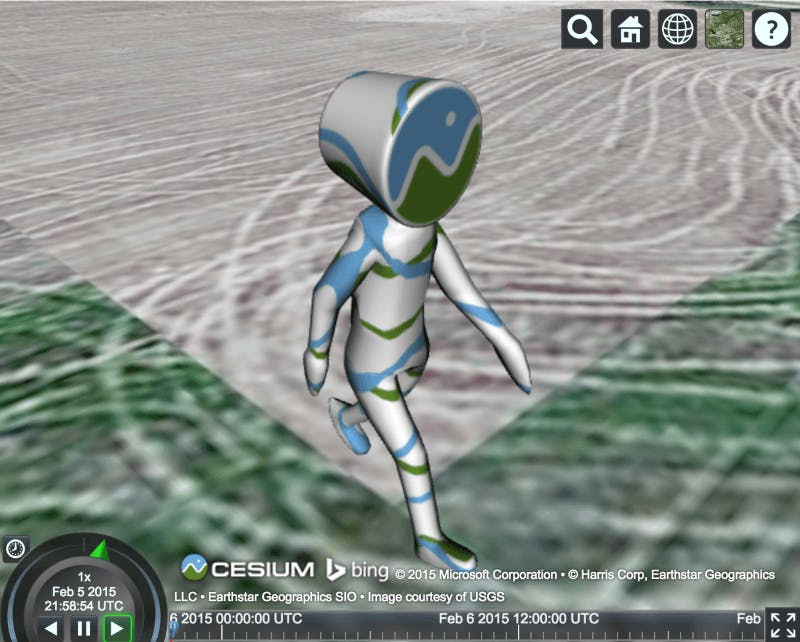
Using terrain
Cesium supports streaming high-resolution global terrain using open-formats including the optimized quantized-mesh format. A global high-resolution terrain data, Cesium World Terrain, hosted by Cesium ion.
Use it in Cesium like this:
viewer.terrainProvider = Cesium.createWorldTerrain();
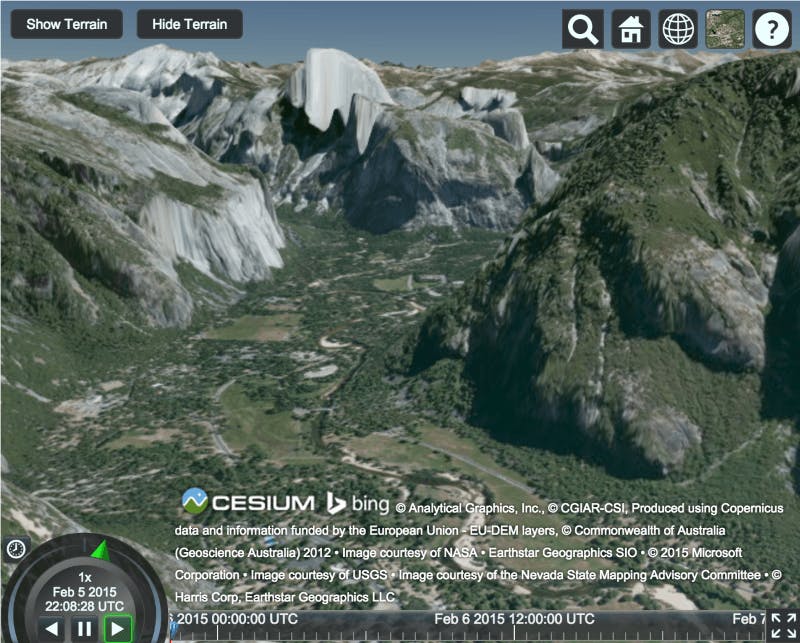
Screen overlays
Since Cesium is not a plugin, other HTML elements can be added over top of it. This is useful for overlaying web UIs and screen overlays.
KML
Initial KML support, including KMZ, was released with Cesium 1.7 on March 2nd, 2015. Check out the live demo in Sandcastle. A good portion of KML 2.2 is supported and future versions of Cesium will continue to add additional capabilities until we reach full parity with Google Earth.
Many users also convert their KML to the Cesium Language, CZML, a JSON schema for describing Cesium scenes. This has many benefits including being web-friendly JSON, first-class support for time-dynamic visualization, and incremental streaming.
Next Steps
Cesium has a lot more to offer. Check out all the Cesium features and the tutorials.