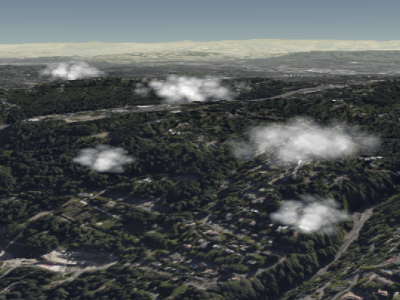
Example cumulus clouds
Clouds are added and removed from the collection using
CloudCollection#add
and CloudCollection#remove
.
Name | Type | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
object |
optional
Object with the following properties:
|
Example:
// Create a cloud collection with two cumulus clouds
const clouds = scene.primitives.add(new Cesium.CloudCollection());
clouds.add({
position : new Cesium.Cartesian3(1.0, 2.0, 3.0),
maximumSize: new Cesium.Cartesian3(20.0, 12.0, 8.0)
});
clouds.add({
position : new Cesium.Cartesian3(4.0, 5.0, 6.0),
maximumSize: new Cesium.Cartesian3(15.0, 9.0, 9.0),
slice: 0.5
});
Demo:
See:
Members
Renders the billboards with one opaque color for the sake of debugging.
-
Default Value:
false
Draws the clouds as opaque, monochrome ellipsoids for the sake of debugging.
If debugBillboards
is also true, then the ellipsoids will draw on top of the billboards.
-
Default Value:
false
Controls the amount of detail captured in the precomputed noise texture
used to render the cumulus clouds. In order for the texture to be tileable,
this must be a power of two. For best results, set this to be a power of two
between 8.0
and 32.0
(inclusive).
clouds.noiseDetail = 8.0; ![]() |
clouds.noiseDetail = 32.0; ![]() |
-
Default Value:
16.0
noiseOffset : Cartesian3
Applies a translation to noise texture coordinates to generate different data. This can be modified if the default noise does not generate good-looking clouds.
default ![]() |
clouds.noiseOffset = new Cesium.Cartesian3(10, 20, 10); ![]() |
-
Default Value:
Cartesian3.ZERO
-
Default Value:
true
Methods
add(options) → CumulusCloud
Performance:
Calling add
is expected constant time. However, the collection's vertex buffer
is rewritten - an O(n)
operation that also incurs CPU to GPU overhead. For
best performance, add as many clouds as possible before calling update
.
Name | Type | Description |
---|---|---|
options |
object | optional A template describing the cloud's properties as shown in Example 1. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Examples:
// Example 1: Add a cumulus cloud, specifying all the default values.
const c = clouds.add({
show : true,
position : Cesium.Cartesian3.ZERO,
scale : new Cesium.Cartesian2(20.0, 12.0),
maximumSize: new Cesium.Cartesian3(20.0, 12.0, 12.0),
slice: -1.0,
cloudType : CloudType.CUMULUS
});
// Example 2: Specify only the cloud's cartographic position.
const c = clouds.add({
position : Cesium.Cartesian3.fromDegrees(longitude, latitude, height)
});
See:
Name | Type | Description |
---|---|---|
cloud |
CumulusCloud | optional The cloud to check for. |
Returns:
See:
Once an object is destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception. Therefore,
assign the return value (undefined
) to the object as done in the example.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
clouds = clouds && clouds.destroy();
See:
get(index) → CumulusCloud
CloudCollection#length
to iterate over all the clouds in the collection.
Performance:
Expected constant time. If clouds were removed from the collection and
CloudCollection#update
was not called, an implicit O(n)
operation is performed.
Name | Type | Description |
---|---|---|
index |
number | The zero-based index of the cloud. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Toggle the show property of every cloud in the collection
const len = clouds.length;
for (let i = 0; i < len; ++i) {
const c = clouds.get(i);
c.show = !c.show;
}
See:
If this object was destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception.
Returns:
true
if this object was destroyed; otherwise, false
.
Name | Type | Description |
---|---|---|
cloud |
CumulusCloud | The cloud to remove. |
Returns:
true
if the cloud was removed; false
if the cloud was not found in the collection.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
const c = clouds.add(...);
clouds.remove(c); // Returns true
See:
Performance:
O(n)
. It is more efficient to remove all the clouds
from a collection and then add new ones than to create a new collection entirely.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
clouds.add(...);
clouds.add(...);
clouds.removeAll();