new BaseLayerPicker(container, options)
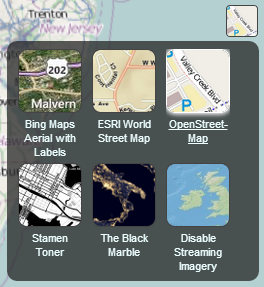
BaseLayerPicker with its drop-panel open.
The BaseLayerPicker is a single button widget that displays a panel of available imagery and terrain providers. When imagery is selected, the corresponding imagery layer is created and inserted as the base layer of the imagery collection; removing the existing base. When terrain is selected, it replaces the current terrain provider. Each item in the available providers list contains a name, a representative icon, and a tooltip to display more information when hovered. The list is initially empty, and must be configured before use, as illustrated in the below example.
Name | Type | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
container |
Element | The parent HTML container node for this widget. | ||||||||||||||||||||||||
options |
Object |
Object with the following properties:
|
Throws:
-
DeveloperError : Element with id "container" does not exist in the document.
Example:
// In HTML head, include a link to the BaseLayerPicker.css stylesheet,
// and in the body, include: <div id="baseLayerPickerContainer"
// style="position:absolute;top:24px;right:24px;width:38px;height:38px;"></div>
//Create the list of available providers we would like the user to select from.
//This example uses 3, OpenStreetMap, The Black Marble, and a single, non-streaming world image.
var imageryViewModels = [];
imageryViewModels.push(new Cesium.ProviderViewModel({
name : 'Open\u00adStreet\u00adMap',
iconUrl : Cesium.buildModuleUrl('Widgets/Images/ImageryProviders/openStreetMap.png'),
tooltip : 'OpenStreetMap (OSM) is a collaborative project to create a free editable \
map of the world.\nhttp://www.openstreetmap.org',
creationFunction : function() {
return new Cesium.OpenStreetMapImageryProvider({
url : '//a.tile.openstreetmap.org/'
});
}
}));
imageryViewModels.push(new Cesium.ProviderViewModel({
name : 'Black Marble',
iconUrl : Cesium.buildModuleUrl('Widgets/Images/ImageryProviders/blackMarble.png'),
tooltip : 'The lights of cities and villages trace the outlines of civilization \
in this global view of the Earth at night as seen by NASA/NOAA\'s Suomi NPP satellite.',
creationFunction : function() {
return new Cesium.TileMapServiceImageryProvider({
url : '//cesiumjs.org/blackmarble',
maximumLevel : 8,
credit : 'Black Marble imagery courtesy NASA Earth Observatory'
});
}
}));
imageryViewModels.push(new Cesium.ProviderViewModel({
name : 'Natural Earth\u00a0II',
iconUrl : Cesium.buildModuleUrl('Widgets/Images/ImageryProviders/naturalEarthII.png'),
tooltip : 'Natural Earth II, darkened for contrast.\nhttp://www.naturalearthdata.com/',
creationFunction : function() {
return new Cesium.TileMapServiceImageryProvider({
url : Cesium.buildModuleUrl('Assets/Textures/NaturalEarthII')
});
}
}));
//Create a CesiumWidget without imagery, if you haven't already done so.
var cesiumWidget = new Cesium.CesiumWidget('cesiumContainer', { imageryProvider: false });
//Finally, create the baseLayerPicker widget using our view models.
var layers = cesiumWidget.scene.imageryLayers;
var baseLayerPicker = new Cesium.BaseLayerPicker('baseLayerPickerContainer', layers, imageryViewModels);
//Use the first item in the list as the current selection.
baseLayerPicker.viewModel.selectedItem = imageryViewModels[0];
See:
Members
-
container :Element
-
Gets the parent container.
-
viewModel :BaseLayerPickerViewModel
-
Gets the view model.
Methods
-
destroy()
-
Destroys the widget. Should be called if permanently removing the widget from layout.
-
isDestroyed() → Boolean
-
Returns:
true if the object has been destroyed, false otherwise.