new BillboardCollection(options)
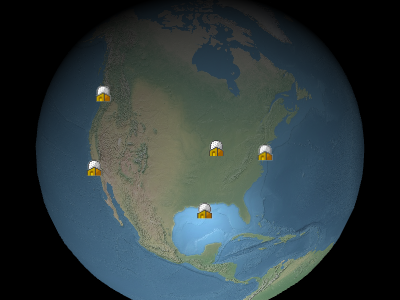
Example billboards
Billboards are added and removed from the collection using
BillboardCollection#add
and BillboardCollection#remove
. Billboards in a collection automatically share textures
for images with the same identifier.
Performance:
For best performance, prefer a few collections, each with many billboards, to many collections with only a few billboards each. Organize collections so that billboards with the same update frequency are in the same collection, i.e., billboards that do not change should be in one collection; billboards that change every frame should be in another collection; and so on.
Name | Type | Description | ||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
Object |
optional
Object with the following properties:
|
Example:
// Create a billboard collection with two billboards
var billboards = scene.primitives.add(new Cesium.BillboardCollection());
billboards.add({
position : new Cesium.Cartesian3(1.0, 2.0, 3.0),
image : 'url/to/image'
});
billboards.add({
position : new Cesium.Cartesian3(4.0, 5.0, 6.0),
image : 'url/to/another/image'
});
Demo:
See:
Members
-
debugShowBoundingVolume :Boolean
-
This property is for debugging only; it is not for production use nor is it optimized.
Draws the bounding sphere for each draw command in the primitive.
-
Default Value:
false
-
length :Number
-
Returns the number of billboards in this collection. This is commonly used with
BillboardCollection#get
to iterate over all the billboards in the collection. -
modelMatrix :Matrix4
-
The 4x4 transformation matrix that transforms each billboard in this collection from model to world coordinates. When this is the identity matrix, the billboards are drawn in world coordinates, i.e., Earth's WGS84 coordinates. Local reference frames can be used by providing a different transformation matrix, like that returned by
Transforms.eastNorthUpToFixedFrame
.-
Default Value:
Matrix4.IDENTITY
Example:
var center = Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883); billboards.modelMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center); billboards.add({ image : 'url/to/image', position : new Cesium.Cartesian3(0.0, 0.0, 0.0) // center }); billboards.add({ image : 'url/to/image', position : new Cesium.Cartesian3(1000000.0, 0.0, 0.0) // east }); billboards.add({ image : 'url/to/image', position : new Cesium.Cartesian3(0.0, 1000000.0, 0.0) // north }); billboards.add({ image : 'url/to/image', position : new Cesium.Cartesian3(0.0, 0.0, 1000000.0) // up });
See:
Methods
-
add(billboard) → Billboard
-
Creates and adds a billboard with the specified initial properties to the collection. The added billboard is returned so it can be modified or removed from the collection later.
Performance:
Calling
add
is expected constant time. However, the collection's vertex buffer is rewritten - anO(n)
operation that also incurs CPU to GPU overhead. For best performance, add as many billboards as possible before callingupdate
.Name Type Description billboard
Object optional A template describing the billboard's properties as shown in Example 1. Returns:
The billboard that was added to the collection.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Examples:
// Example 1: Add a billboard, specifying all the default values. var b = billboards.add({ show : true, position : Cesium.Cartesian3.ZERO, pixelOffset : Cesium.Cartesian2.ZERO, eyeOffset : Cesium.Cartesian3.ZERO, horizontalOrigin : Cesium.HorizontalOrigin.CENTER, verticalOrigin : Cesium.VerticalOrigin.CENTER, scale : 1.0, image : 'url/to/image', color : Cesium.Color.WHITE, id : undefined });
// Example 2: Specify only the billboard's cartographic position. var b = billboards.add({ position : Cesium.Cartesian3.fromDegrees(longitude, latitude, height) });
See:
-
-
contains(billboard) → Boolean
-
Check whether this collection contains a given billboard.
Name Type Description billboard
Billboard optional The billboard to check for. Returns:
true if this collection contains the billboard, false otherwise. -
destroy() → undefined
-
Destroys the WebGL resources held by this object. Destroying an object allows for deterministic release of WebGL resources, instead of relying on the garbage collector to destroy this object.
Once an object is destroyed, it should not be used; calling any function other thanisDestroyed
will result in aDeveloperError
exception. Therefore, assign the return value (undefined
) to the object as done in the example.Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
billboards = billboards && billboards.destroy();
See:
-
-
get(index) → Billboard
-
Returns the billboard in the collection at the specified index. Indices are zero-based and increase as billboards are added. Removing a billboard shifts all billboards after it to the left, changing their indices. This function is commonly used with
BillboardCollection#length
to iterate over all the billboards in the collection.Performance:
Expected constant time. If billboards were removed from the collection and
BillboardCollection#update
was not called, an implicitO(n)
operation is performed.Name Type Description index
Number The zero-based index of the billboard. Returns:
The billboard at the specified index.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Toggle the show property of every billboard in the collection var len = billboards.length; for (var i = 0; i < len; ++i) { var b = billboards.get(i); b.show = !b.show; }
See:
-
-
isDestroyed() → Boolean
-
Returns true if this object was destroyed; otherwise, false.
If this object was destroyed, it should not be used; calling any function other thanisDestroyed
will result in aDeveloperError
exception.Returns:
true
if this object was destroyed; otherwise,false
. -
remove(billboard) → Boolean
-
Removes a billboard from the collection.
Performance:
Calling
remove
is expected constant time. However, the collection's vertex buffer is rewritten - anO(n)
operation that also incurs CPU to GPU overhead. For best performance, remove as many billboards as possible before callingupdate
. If you intend to temporarily hide a billboard, it is usually more efficient to callBillboard#show
instead of removing and re-adding the billboard.Name Type Description billboard
Billboard The billboard to remove. Returns:
true
if the billboard was removed;false
if the billboard was not found in the collection.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
var b = billboards.add(...); billboards.remove(b); // Returns true
See:
-
-
removeAll()
-
Removes all billboards from the collection.
Performance:
O(n)
. It is more efficient to remove all the billboards from a collection and then add new ones than to create a new collection entirely.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
billboards.add(...); billboards.add(...); billboards.removeAll();
See:
-
-
update()
-
Called when
Viewer
orCesiumWidget
render the scene to get the draw commands needed to render this primitive.Do not call this function directly. This is documented just to list the exceptions that may be propagated when the scene is rendered:
Throws:
-
RuntimeError : image with id must be in the atlas.
-