new Billboard()
BillboardCollection
. A billboard is created and its initial
properties are set by calling BillboardCollection#add
.
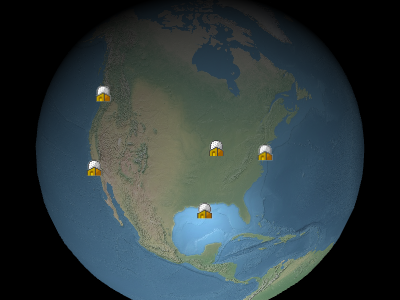
Example billboards
Performance:
Reading a property, e.g., Billboard#show
, is constant time.
Assigning to a property is constant time but results in
CPU to GPU traffic when BillboardCollection#update
is called. The per-billboard traffic is
the same regardless of how many properties were updated. If most billboards in a collection need to be
updated, it may be more efficient to clear the collection with BillboardCollection#removeAll
and add new billboards instead of modifying each one.
Throws:
-
DeveloperError : scaleByDistance.far must be greater than scaleByDistance.near
-
DeveloperError : translucencyByDistance.far must be greater than translucencyByDistance.near
-
DeveloperError : pixelOffsetScaleByDistance.far must be greater than pixelOffsetScaleByDistance.near
Members
-
alignedAxis :Cartesian3
-
Gets or sets the aligned axis in world space. The aligned axis is the unit vector that the billboard up vector points towards. The default is the zero vector, which means the billboard is aligned to the screen up vector.
Examples:
// Example 1. // Have the billboard up vector point north billboard.alignedAxis = Cesium.Cartesian3.UNIT_Z;
// Example 2. // Have the billboard point east. billboard.alignedAxis = Cartesian3.UNIT_Z; billboard.rotation = -Cesium.Math.PI_OVER_TWO;
// Example 3. // Reset the aligned axis billboard.alignedAxis = Cesium.Cartesian3.ZERO;
Source: Scene/Billboard.js, line 644 -
color
-
Gets or sets the color that is multiplied with the billboard's texture. This has two common use cases. First, the same white texture may be used by many different billboards, each with a different color, to create colored billboards. Second, the color's alpha component can be used to make the billboard translucent as shown below. An alpha of
0.0
makes the billboard transparent, and1.0
makes the billboard opaque.
default
alpha : 0.5
The red, green, blue, and alpha values are indicated byvalue
'sred
,green
,blue
, andalpha
properties as shown in Example 1. These components range from0.0
(no intensity) to1.0
(full intensity).Examples:
// Example 1. Assign yellow. b.color = Cesium.Color.YELLOW;
// Example 2. Make a billboard 50% translucent. b.color = new Cesium.Color(1.0, 1.0, 1.0, 0.5);
Source: Scene/Billboard.js, line 581 -
eyeOffset :Cartesian3
-
Gets or sets the 3D Cartesian offset applied to this billboard in eye coordinates. Eye coordinates is a left-handed coordinate system, where
x
points towards the viewer's right,y
points up, andz
points into the screen. Eye coordinates use the same scale as world and model coordinates, which is typically meters.
An eye offset is commonly used to arrange multiple billboards or objects at the same position, e.g., to arrange a billboard above its corresponding 3D model.
Below, the billboard is positioned at the center of the Earth but an eye offset makes it always appear on top of the Earth regardless of the viewer's or Earth's orientation.
b.eyeOffset = new Cartesian3(0.0, 8000000.0, 0.0);
Source: Scene/Billboard.js, line 439 -
height :Number
-
Gets or sets a height for the billboard. If undefined, the image height will be used.Source: Scene/Billboard.js, line 685
-
heightReference :HeightReference
-
Gets or sets the height reference of this billboard.
-
Default Value:
HeightReference.NONE
Source: Scene/Billboard.js, line 238 -
horizontalOrigin :HorizontalOrigin
-
Gets or sets the horizontal origin of this billboard, which determines if the billboard is to the left, center, or right of its position.
Example:
// Use a bottom, left origin b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT; b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
Source: Scene/Billboard.js, line 472 -
id :Object
-
Gets or sets the user-defined object returned when the billboard is picked.Source: Scene/Billboard.js, line 702
-
image :String
-
Gets or sets the image to be used for this billboard. If a texture has already been created for the given image, the existing texture is used.
This property can be set to a loaded Image, a URL which will be loaded as an Image automatically, a canvas, or another billboard's image property (from the same billboard collection).
Example:
// load an image from a URL b.image = 'some/image/url.png'; // assuming b1 and b2 are billboards in the same billboard collection, // use the same image for both billboards. b2.image = b1.image;
Source: Scene/Billboard.js, line 751 -
pixelOffset :Cartesian2
-
Gets or sets the pixel offset in screen space from the origin of this billboard. This is commonly used to align multiple billboards and labels at the same position, e.g., an image and text. The screen space origin is the top, left corner of the canvas;
x
increases from left to right, andy
increases from top to bottom.
The billboard's origin is indicated by the yellow point.default
b.pixeloffset = new Cartesian2(50, 25);
Source: Scene/Billboard.js, line 274 -
pixelOffsetScaleByDistance :NearFarScalar
-
Gets or sets near and far pixel offset scaling properties of a Billboard based on the billboard's distance from the camera. A billboard's pixel offset will be scaled between the
NearFarScalar#nearValue
andNearFarScalar#farValue
while the camera distance falls within the upper and lower bounds of the specifiedNearFarScalar#near
andNearFarScalar#far
. Outside of these ranges the billboard's pixel offset scale remains clamped to the nearest bound. If undefined, pixelOffsetScaleByDistance will be disabled.Examples:
// Example 1. // Set a billboard's pixel offset scale to 0.0 when the // camera is 1500 meters from the billboard and scale pixel offset to 10.0 pixels // in the y direction the camera distance approaches 8.0e6 meters. b.pixelOffset = new Cesium.Cartesian2(0.0, 1.0); b.pixelOffsetScaleByDistance = new Cesium.NearFarScalar(1.5e2, 0.0, 8.0e6, 10.0);
// Example 2. // disable pixel offset by distance b.pixelOffsetScaleByDistance = undefined;
Source: Scene/Billboard.js, line 398 -
position :Cartesian3
-
Gets or sets the Cartesian position of this billboard.Source: Scene/Billboard.js, line 210
-
readonlyready :Boolean
-
When
true
, this billboard is ready to render, i.e., the image has been downloaded and the WebGL resources are created.-
Default Value:
false
Source: Scene/Billboard.js, line 784 -
rotation :Number
-
Gets or sets the rotation angle in radians.Source: Scene/Billboard.js, line 605
-
scale :Number
-
Gets or sets the uniform scale that is multiplied with the billboard's image size in pixels. A scale of
1.0
does not change the size of the billboard; a scale greater than1.0
enlarges the billboard; a positive scale less than1.0
shrinks the billboard.
From left to right in the above image, the scales are0.5
,1.0
, and2.0
.Source: Scene/Billboard.js, line 536 -
scaleByDistance :NearFarScalar
-
Gets or sets near and far scaling properties of a Billboard based on the billboard's distance from the camera. A billboard's scale will interpolate between the
NearFarScalar#nearValue
andNearFarScalar#farValue
while the camera distance falls within the upper and lower bounds of the specifiedNearFarScalar#near
andNearFarScalar#far
. Outside of these ranges the billboard's scale remains clamped to the nearest bound. If undefined, scaleByDistance will be disabled.Examples:
// Example 1. // Set a billboard's scaleByDistance to scale by 1.5 when the // camera is 1500 meters from the billboard and disappear as // the camera distance approaches 8.0e6 meters. b.scaleByDistance = new Cesium.NearFarScalar(1.5e2, 1.5, 8.0e6, 0.0);
// Example 2. // disable scaling by distance b.scaleByDistance = undefined;
Source: Scene/Billboard.js, line 315 -
show :Boolean
-
Determines if this billboard will be shown. Use this to hide or show a billboard, instead of removing it and re-adding it to the collection.Source: Scene/Billboard.js, line 187
-
translucencyByDistance :NearFarScalar
-
Gets or sets near and far translucency properties of a Billboard based on the billboard's distance from the camera. A billboard's translucency will interpolate between the
NearFarScalar#nearValue
andNearFarScalar#farValue
while the camera distance falls within the upper and lower bounds of the specifiedNearFarScalar#near
andNearFarScalar#far
. Outside of these ranges the billboard's translucency remains clamped to the nearest bound. If undefined, translucencyByDistance will be disabled.Examples:
// Example 1. // Set a billboard's translucency to 1.0 when the // camera is 1500 meters from the billboard and disappear as // the camera distance approaches 8.0e6 meters. b.translucencyByDistance = new Cesium.NearFarScalar(1.5e2, 1.0, 8.0e6, 0.0);
// Example 2. // disable translucency by distance b.translucencyByDistance = undefined;
Source: Scene/Billboard.js, line 356 -
verticalOrigin :VerticalOrigin
-
Gets or sets the vertical origin of this billboard, which determines if the billboard is to the above, below, or at the center of its position.
Example:
// Use a bottom, left origin b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT; b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
Source: Scene/Billboard.js, line 504 -
width :Number
-
Gets or sets a width for the billboard. If undefined, the image width will be used.Source: Scene/Billboard.js, line 668
Methods
-
computeScreenSpacePosition(scene, result) → Cartesian2
-
Computes the screen-space position of the billboard's origin, taking into account eye and pixel offsets. The screen space origin is the top, left corner of the canvas;
x
increases from left to right, andy
increases from top to bottom.Name Type Description scene
Scene The scene. result
Cartesian2 optional The object onto which to store the result. Returns:
The screen-space position of the billboard.Throws:
-
DeveloperError : Billboard must be in a collection.
Example:
console.log(b.computeScreenSpacePosition(scene).toString());
See:
Source: Scene/Billboard.js, line 1122 -
-
equals(other) → Boolean
-
Determines if this billboard equals another billboard. Billboards are equal if all their properties are equal. Billboards in different collections can be equal.
Name Type Description other
Billboard The billboard to compare for equality. Returns:
true
if the billboards are equal; otherwise,false
.Source: Scene/Billboard.js, line 1157 -
setImage(id, image)
-
Sets the image to be used for this billboard. If a texture has already been created for the given id, the existing texture is used.
This function is useful for dynamically creating textures that are shared across many billboards. Only the first billboard will actually call the function and create the texture, while subsequent billboards created with the same id will simply re-use the existing texture.
To load an image from a URL, setting the
Billboard#image
property is more convenient.Name Type Description id
String The id of the image. This can be any string that uniquely identifies the image. image
Image | Canvas | String | Billboard~CreateImageCallback The image to load. This parameter can either be a loaded Image or Canvas, a URL which will be loaded as an Image automatically, or a function which will be called to create the image if it hasn't been loaded already. Example:
// create a billboard image dynamically function drawImage(id) { // create and draw an image using a canvas var canvas = document.createElement('canvas'); var context2D = canvas.getContext('2d'); // ... draw image return canvas; } // drawImage will be called to create the texture b.setImage('myImage', drawImage); // subsequent billboards created in the same collection using the same id will use the existing // texture, without the need to create the canvas or draw the image b2.setImage('myImage', drawImage);
Source: Scene/Billboard.js, line 973 -
setImageSubRegion(id, subRegion)
-
Uses a sub-region of the image with the given id as the image for this billboard.
Name Type Description id
String The id of the image to use. subRegion
BoundingRectangle The sub-region of the image. Throws:
-
RuntimeError : image with id must be in the atlas
Source: Scene/Billboard.js, line 1005 -
Type Definitions
-
CreateImageCallback(id) → Image|Canvas|Promise.<(Image|Canvas)>
-
A function that creates an image.
Name Type Description id
String The identifier of the image to load. Returns:
The image, or a promise that will resolve to an image.Source: Scene/Billboard.js, line 1188