new Billboard
A viewport-aligned image positioned in the 3D scene, that is created
and rendered using a BillboardCollection. A billboard is created and its initial
properties are set by calling BillboardCollection#add. Any of the billboard's
properties can be changed at any time by calling the billboard's corresponding
set
function, e.g., Billboard#setShow.
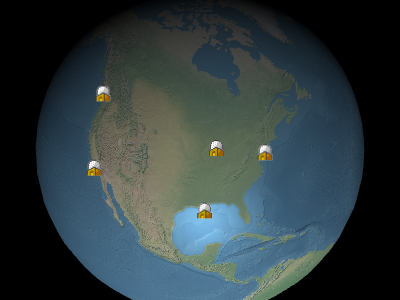
Example billboards
Performance:
Calling any get
function, e.g., Billboard#getShow, is constant time.
Calling a set
function, e.g., Billboard#setShow, is constant time but results in
CPU to GPU traffic when BillboardCollection#update is called. The per-billboard traffic is
the same regardless of how many properties were updated. If most billboards in a collection need to be
updated, it may be more efficient to clear the collection with BillboardCollection#removeAll
and add new billboards instead of modifying each one.
Methods
-
computeScreenSpacePosition
-
Computes the screen-space position of the billboard's origin, taking into account eye and pixel offsets. The screen space origin is the bottom, left corner of the canvas;
x
increases from left to right, andy
increases from bottom to top.Parameters:
Name Type Description context
Context The context. frameState
FrameState The same state object passed to BillboardCollection#update. Throws:
-
DeveloperError : Billboard must be in a collection.
-
DeveloperError : context is required.
-
DeveloperError : frameState is required.
Returns:
Cartesian2 The screen-space position of the billboard.Example
console.log(b.computeScreenSpacePosition(scene.getContext(), scene.getFrameState()).toString());
-
-
equals
-
Determines if this billboard equals another billboard. Billboards are equal if all their properties are equal. Billboards in different collections can be equal.
Parameters:
Name Type Description other
Billboard The billboard to compare for equality. Returns:
Booleantrue
if the billboards are equal; otherwise,false
. -
getColor
-
Returns the color that is multiplied with the billboard's texture. The red, green, blue, and alpha values are indicated by the returned object's
red
,green
,blue
, andalpha
properties, which range from0
(no intensity) to1.0
(full intensity).Returns:
Number The color that is multiplied with the billboard's texture.See:
-
getEyeOffset
-
Returns the 3D Cartesian offset applied to this billboard in eye coordinates.
Returns:
Cartesian3 The 3D Cartesian offset applied to this billboard in eye coordinates. -
getHorizontalOrigin
-
Returns the horizontal origin of this billboard.
Returns:
HorizontalOrigin The horizontal origin of this billboard. -
getImageIndex
-
DOC_TBA
-
getPixelOffset
-
Returns the pixel offset from the origin of this billboard.
Returns:
Cartesian2 The pixel offset of this billboard. -
getPosition
-
Returns the Cartesian position of this billboard.
Returns:
Cartesian3 The Cartesian position of this billboard. -
getScale
-
Returns the uniform scale that is multiplied with the billboard's image size in pixels.
Returns:
Number The scale used to size the billboard.See:
-
getShow
-
Returns true if this billboard will be shown. Call Billboard#setShow to hide or show a billboard, instead of removing it and re-adding it to the collection.
Returns:
Booleantrue
if this billboard will be shown; otherwise,false
.See:
-
getVerticalOrigin
-
Returns the vertical origin of this billboard.
Returns:
VerticalOrigin The vertical origin of this billboard. -
setColor
-
Sets the color that is multiplied with the billboard's texture. This has two common use cases. First, the same white texture may be used by many different billboards, each with a different color, to create colored billboards. Second, the color's alpha component can be used to make the billboard translucent as shown below. An alpha of
0.0
makes the billboard transparent, and1.0
makes the billboard opaque.
default
alpha : 0.5
The red, green, blue, and alpha values are indicated byvalue
'sred
,green
,blue
, andalpha
properties as shown in Example 1. These components range from0.0
(no intensity) to1.0
(full intensity).Parameters:
Name Type Description value
Object The color's red, green, blue, and alpha components. Throws:
DeveloperError : value is required.Example
// Example 1. Assign yellow. b.setColor({ red : 1.0, green : 1.0, blue : 0.0, alpha : 1.0 }); // Example 2. Make a billboard 50% translucent. b.setColor({ red : 1.0, green : 1.0, blue : 1.0, alpha : 0.5 });
See:
-
setEyeOffset
-
Sets the 3D Cartesian offset applied to this billboard in eye coordinates. Eye coordinates is a left-handed coordinate system, where
x
points towards the viewer's right,y
points up, andz
points into the screen. Eye coordinates use the same scale as world and model coordinates, which is typically meters.
An eye offset is commonly used to arrange multiple billboards or objects at the same position, e.g., to arrange a billboard above its corresponding 3D model.
value
can be either a Cartesian3 or an object literal withx
,y
, andz
properties. A copy ofvalue
is made, so changing it after callingsetEyeOffset
does not affect the billboard's eye offset; an explicit call tosetEyeOffset
is required.
Below, the billboard is positioned at the center of the Earth but an eye offset makes it always appear on top of the Earth regardless of the viewer's or Earth's orientation.
b.setEyeOffset({ x : 0.0, y : 8000000.0, z : 0.0 });
Parameters:
Name Type Description value
Cartesian3 The 3D Cartesian offset in eye coordinates. Throws:
DeveloperError : value is required. -
setHorizontalOrigin
-
Sets the horizontal origin of this billboard, which determines if the billboard is to the left, center, or right of its position.
Parameters:
Name Type Description value
HorizontalOrigin The horizontal origin. Throws:
DeveloperError : value is required.Example
// Use a bottom, left origin b.setHorizontalOrigin(HorizontalOrigin.LEFT); b.setVerticalOrigin(VerticalOrigin.BOTTOM);
-
setImageIndex
-
DOC_TBA
-
setPixelOffset
-
Sets the pixel offset in screen space from the origin of this billboard. This is commonly used to align multiple billboards and labels at the same position, e.g., an image and text. The screen space origin is the bottom, left corner of the canvas;
x
increases from left to right, andy
increases from bottom to top.
value
can be either a Cartesian2 or an object literal withx
andy
properties. A copy ofvalue
is made, so changing it after callingsetPixelOffset
does not affect the billboard's pixel offset; an explicit call tosetPixelOffset
is required.
The billboard's origin is indicated by the yellow point.default
b.setPixelOffset({ x : 50, y : -25 });
Parameters:
Name Type Description value
Cartesian2 The 2D Cartesian pixel offset. Throws:
DeveloperError : value is required. -
setPosition
-
Sets the Cartesian position of this billboard.
As shown in the examples,value
can be either a Cartesian3 or an object literal withx
,y
, andz
properties. A copy ofvalue
is made, so changing it after callingsetPosition
does not affect the billboard's position; an explicit call tosetPosition
is required.Parameters:
Name Type Description value
Cartesian3 The Cartesian position. Throws:
DeveloperError : value is required.Example
// Example 1. Set a billboard's position using a Cartesian3. b.setPosition(new Cartesian3(1.0, 2.0, 3.0)); // Example 2. Set a billboard's position using an object literal. b.setPosition({ x : 1.0, y : 2.0, z : 3.0 });
-
setScale
-
Sets the uniform scale that is multiplied with the billboard's image size in pixels. A scale of
1.0
does not change the size of the billboard; a scale greater than1.0
enlarges the billboard; a positive scale less than1.0
shrinks the billboard.
From left to right in the above image, the scales are0.5
,1.0
, and2.0
.Parameters:
Name Type Description value
Number The scale used to size the billboard. Throws:
DeveloperError : value is required. -
setShow
-
Determines if this billboard will be shown. Call this to hide or show a billboard, instead of removing it and re-adding it to the collection.
Parameters:
Name Type Description value
Boolean Indicates if this billboard will be shown. Throws:
DeveloperError : value is required.See:
-
setVerticalOrigin
-
Sets the vertical origin of this billboard, which determines if the billboard is to the above, below, or at the center of its position.
Parameters:
Name Type Description value
VerticalOrigin The vertical origin. Throws:
DeveloperError : value is required.Example
// Use a bottom, left origin b.setHorizontalOrigin(HorizontalOrigin.LEFT); b.setVerticalOrigin(VerticalOrigin.BOTTOM);