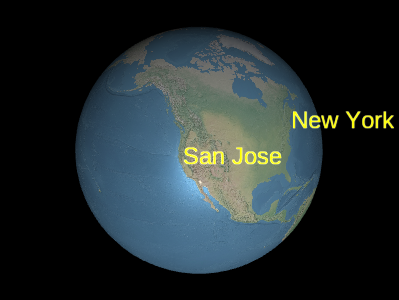
Example labels
Labels are added and removed from the collection using
LabelCollection#add
and LabelCollection#remove
.
Performance:
For best performance, prefer a few collections, each with many labels, to many collections with only a few labels each. Avoid having collections where some labels change every frame and others do not; instead, create one or more collections for static labels, and one or more collections for dynamic labels.
Name | Type | Description | ||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
Object |
optional
Object with the following properties:
|
Example:
// Create a label collection with two labels
var labels = scene.primitives.add(new Cesium.LabelCollection());
labels.add({
position : new Cesium.Cartesian3(1.0, 2.0, 3.0),
text : 'A label'
});
labels.add({
position : new Cesium.Cartesian3(4.0, 5.0, 6.0),
text : 'Another label'
});
Demo:
See:
Members
-
This property is for debugging only; it is not for production use nor is it optimized.
Draws the bounding sphere for each draw command in the primitive.
-
Default Value:
false
-
Returns the number of labels in this collection. This is commonly used with
LabelCollection#get
to iterate over all the labels in the collection. -
modelMatrix : Matrix4
-
The 4x4 transformation matrix that transforms each label in this collection from model to world coordinates. When this is the identity matrix, the labels are drawn in world coordinates, i.e., Earth's WGS84 coordinates. Local reference frames can be used by providing a different transformation matrix, like that returned by
Transforms.eastNorthUpToFixedFrame
.-
Default Value:
Matrix4.IDENTITY
Example:
var center = Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883); labels.modelMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center); labels.add({ position : new Cesium.Cartesian3(0.0, 0.0, 0.0), text : 'Center' }); labels.add({ position : new Cesium.Cartesian3(1000000.0, 0.0, 0.0), text : 'East' }); labels.add({ position : new Cesium.Cartesian3(0.0, 1000000.0, 0.0), text : 'North' }); labels.add({ position : new Cesium.Cartesian3(0.0, 0.0, 1000000.0), text : 'Up' });
Methods
-
add(options) → Label
-
Creates and adds a label with the specified initial properties to the collection. The added label is returned so it can be modified or removed from the collection later.
Performance:
Calling
add
is expected constant time. However, the collection's vertex buffer is rewritten; this operations isO(n)
and also incurs CPU to GPU overhead. For best performance, add as many billboards as possible before callingupdate
.Name Type Description options
Object optional A template describing the label's properties as shown in Example 1. Returns:
The label that was added to the collection.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Examples:
// Example 1: Add a label, specifying all the default values. var l = labels.add({ show : true, position : Cesium.Cartesian3.ZERO, text : '', font : '30px sans-serif', fillColor : Cesium.Color.WHITE, outlineColor : Cesium.Color.BLACK, style : Cesium.LabelStyle.FILL, pixelOffset : Cesium.Cartesian2.ZERO, eyeOffset : Cesium.Cartesian3.ZERO, horizontalOrigin : Cesium.HorizontalOrigin.LEFT, verticalOrigin : Cesium.VerticalOrigin.BOTTOM, scale : 1.0 });
// Example 2: Specify only the label's cartographic position, // text, and font. var l = labels.add({ position : Cesium.Cartesian3.fromRadians(longitude, latitude, height), text : 'Hello World', font : '24px Helvetica', });
See:
-
-
Check whether this collection contains a given label.
Name Type Description label
Label The label to check for. Returns:
true if this collection contains the label, false otherwise.See:
-
Destroys the WebGL resources held by this object. Destroying an object allows for deterministic release of WebGL resources, instead of relying on the garbage collector to destroy this object.
Once an object is destroyed, it should not be used; calling any function other thanisDestroyed
will result in aDeveloperError
exception. Therefore, assign the return value (undefined
) to the object as done in the example.Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
labels = labels && labels.destroy();
See:
-
-
get(index) → Label
-
Returns the label in the collection at the specified index. Indices are zero-based and increase as labels are added. Removing a label shifts all labels after it to the left, changing their indices. This function is commonly used with
LabelCollection#length
to iterate over all the labels in the collection.Performance:
Expected constant time. If labels were removed from the collection and
Scene#render
was not called, an implicitO(n)
operation is performed.Name Type Description index
Number The zero-based index of the billboard. Returns:
The label at the specified index.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Toggle the show property of every label in the collection var len = labels.length; for (var i = 0; i < len; ++i) { var l = billboards.get(i); l.show = !l.show; }
See:
-
-
Returns true if this object was destroyed; otherwise, false.
If this object was destroyed, it should not be used; calling any function other thanisDestroyed
will result in aDeveloperError
exception.Returns:
True if this object was destroyed; otherwise, false. -
Removes a label from the collection. Once removed, a label is no longer usable.
Performance:
Calling
remove
is expected constant time. However, the collection's vertex buffer is rewritten - anO(n)
operation that also incurs CPU to GPU overhead. For best performance, remove as many labels as possible before callingupdate
. If you intend to temporarily hide a label, it is usually more efficient to callLabel#show
instead of removing and re-adding the label.Name Type Description label
Label The label to remove. Returns:
true
if the label was removed;false
if the label was not found in the collection.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
var l = labels.add(...); labels.remove(l); // Returns true
See:
-
-
Removes all labels from the collection.
Performance:
O(n)
. It is more efficient to remove all the labels from a collection and then add new ones than to create a new collection entirely.Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
labels.add(...); labels.add(...); labels.removeAll();
See:
-